Generator Overview¶
The following diagram summarizes Shiboken’s role in the Qt for Python project.
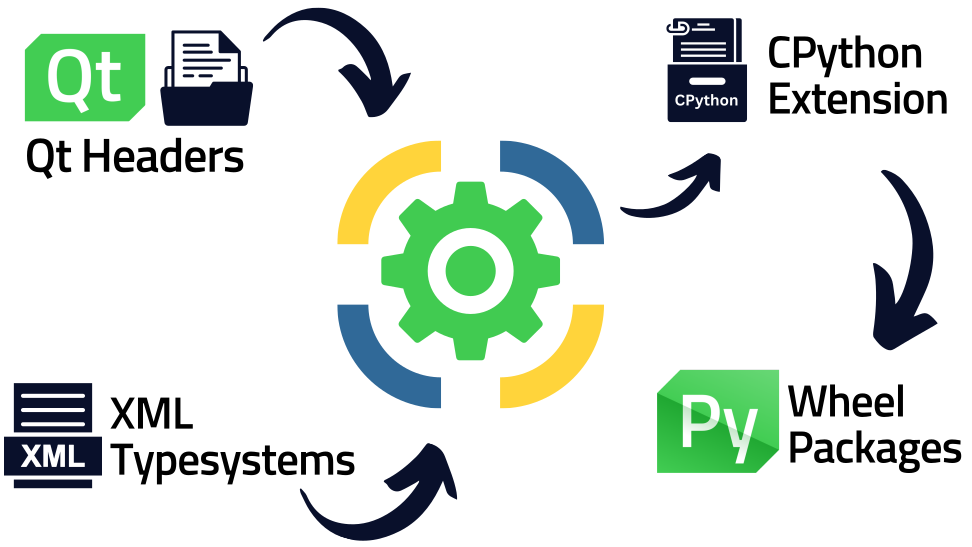
An XML typesystem file is used to specify the types to be exposed to Python and to apply modifications to properly represent and manipulate the types in the Python World. For example, you can remove and add methods to certain types, and also modify the arguments of each method. These actions are inevitable to properly handle the data structures or types.
The final outcome of this process is a set of wrappers written in CPython, which can be used as a module in your Python code.
In a few words, the Generator is a utility that parses a collection of header and typesystem files, generating other files (code, documentation, etc.) as result.
Creating new bindings¶

Creating new bindings¶
Each module of the generator system has an specific role.
Provide enough data about the classes and functions.
Generate valid code, with modifications from typesystems and injected codes.
Modify the API to expose the objects in a way that fits you target language best.
Insert customizations where handwritten code is needed.
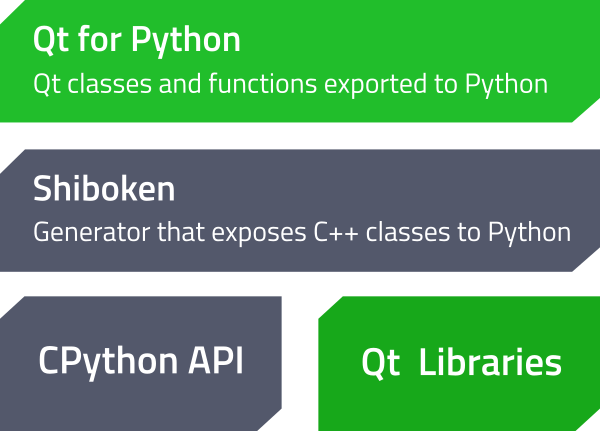
Runtime architecture¶
The newly created binding will run on top of Shiboken which takes care of interfacing Python and the underlying C++ library.
Handwritten inputs¶
Creating new bindings involves creating several pieces of “code”: the header, the typesystem and, in most cases, the injected code.
- header A header with
#include
directives listing all the headers of the desired classes. This header is not referenced by the generated code. Alternatively, it is possible to pass a list of the headers of the desired classes directly on the command line. In this case, the command line option
--use-global-header
should be passed as well to prevent the headers from being suppressed in the generated code.- :typesystem: XML files that provides the developer with a tool to customize the
way that the generators will see the classes and functions. For example, functions can be renamed, have its signature changed and many other actions.
- :inject code: allows the developer to insert
handwritten code where the generated code is not suitable or needs some customization.
Command line options¶
Usage¶
shiboken [options] header-file(s) typesystem-file
Options¶
--disable-verbose-error-messages
Disable verbose error messages. Turn the CPython code hard to debug but saves a few kilobytes in the generated binding.
--enable-parent-ctor-heuristic
This flag enable an useful heuristic which can save a lot of work related to object ownership when writing the typesystem. For more info, check Parentship heuristics.
--enable-pyside-extensions
Enable pyside extensions like support for signal/slots. Use this if you are creating a binding based on PySide.
--enable-return-value-heuristic
Enable heuristics to detect parent relationship on return values. For more info, check Return value heuristics.
--avoid-protected-hack
Avoid the use of the ‘#define protected public’ hack.
--use-isnull-as-nb-bool
If a class has an isNull() const method, it will be used to compute the value of boolean casts (see Bool Cast). The legacy option
--use-isnull-as-nb_nonzero
has the same effect, but should not be used any more.--lean-headers
Forward declare classes in module headers instead of including their class headers where possible.
--use-operator-bool-as-nb-bool
If a class has an operator bool, it will be used to compute the value of boolean casts (see Bool Cast). The legacy option
--use-operator-bool-as-nb_nonzero
has the same effect, but should not be used any more.
--no-implicit-conversions
Do not generate implicit_conversions for function arguments.
--api-version=<version>
Specify the supported api version used to generate the bindings.
--documentation-only
Do not generate any code, just the documentation.
--drop-type-entries="<TypeEntry0>[;TypeEntry1;...]"
Semicolon separated list of type system entries (classes, namespaces, global functions and enums) to be dropped from generation. Values are fully qualified Python type names (‘Module.Class’), but the module can be omitted (‘Class’).
-keywords=keyword1[,keyword2,...]
A comma-separated list of keywords for conditional typesystem parsing (see Conditional Processing).
--use-global-header
Use the global headers passed on the command line in generated code.
--generation-set
Generator set to be used (e.g. qtdoc).
--skip-deprecated
Skip deprecated functions.
--diff
Print a diff of wrapper files.
--dryrun
Dry run, do not generate wrapper files.
--project-file=<file>
Text file containing a description of the binding project. Replaces and overrides command line arguments.
--clang-option=<option>
Option to be passed to clang
--clang-options=<option1>[,<option2>,...]>
Options to be passed to clang. When ‘-’ is passed as the first option in the list, none of the options built into shiboken will be added, allowing for a complete replacement.
--compiler=<type>
Emulated compiler type (g++, msvc, clang)
--compiler-path=<file>
Path to the compiler for determining builtin include paths
--platform=<file>
Emulated platform (windows, darwin, unix)
-I<path>, --include-paths=<path>[:<path>:...]
Include paths used by the C++ parser.
-isystem<path>, --system-include-paths=<path>[:<path>:...]
System include paths used by the C++ parser
-F<path>, --framework-include-paths=<path>[:<path>:...]
Framework include paths used by the C++ parser
--force-process-system-include-paths=<path>[:<path>:...]
Include paths that are considered as system headers by the C++ parser, but should still be processed to extract types
--language-level=, -std=<level>
C++ Language level (c++11..c++17, default=c++14)
-T<path>, --typesystem-paths=<path>[:<path>:...]
Paths used when searching for type system files.
--output-directory=[dir]
The directory where the generated files will be written.
--license-file=[license-file]
File used for copyright headers of generated files.
--no-suppress-warnings
Show all warnings.
--log-unmatched
Prints suppress-warning and rejection elements that were not matched. This is useful for cleaning up old type system files.
--silent
Avoid printing any message.
--debug-level=[sparse|medium|full]
Set the debug level.
--help
Display this help and exit.
--print-builtin-types
Print information about builtin types
--version
Output version information and exit.
QtDocGenerator Options¶
--doc-parser=<parser>
The documentation parser used to interpret the documentation input files (qdoc|doxygen).
--documentation-code-snippets-dir=<dir>
Directory used to search code snippets used by the documentation.
--documentation-data-dir=<dir>
Directory with XML files generated by documentation tool.
--documentation-extra-sections-dir=<dir>
Directory used to search for extra documentation sections.
--library-source-dir=<dir>
Directory where library source code is located.
--additional-documentation=<file>
List of additional XML files to be converted to .rst files (for example, tutorials).
--inheritance-file=<file>
Generate a JSON file containing the class inheritance.
--disable-inheritance-diagram
Disable the generation of the inheritance diagram.
Binding Project File¶
Instead of directing the Generator behavior via command line, the binding developer can write a text project file describing the same information, and avoid the hassle of a long stream of command line arguments.
The project file structure¶
Here follows a comprehensive example of a generator project file.
[generator-project]
generator-set = path/to/generator/CHOICE_GENERATOR
header-file = DIR/global.h" />
typesystem-file = DIR/typesystem_for_your_binding.xml
output-directory location="OUTPUTDIR" />
include-path = path/to/library/being/wrapped/headers/1
include-path = path/to/library/being/wrapped/headers/2
typesystem-path = path/to/directory/containing/type/system/files/1
typesystem-path = path/to/directory/containing/type/system/files/2
enable-parent-ctor-heuristic