QDate¶
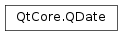
Synopsis¶
Functions¶
def
__eq__
(other)def
__ge__
(other)def
__gt__
(other)def
__le__
(other)def
__lt__
(other)def
__ne__
(other)def
__reduce__
()def
__repr__
()def
addDays
(days)def
addMonths
(months)def
addYears
(years)def
day
()def
dayOfWeek
()def
dayOfYear
()def
daysInMonth
()def
daysInYear
()def
daysTo
(arg__1)def
getDate
(year, month, day)def
isNull
()def
isValid
()def
month
()def
setDate
(year, month, day)def
toJulianDay
()def
toPython
()def
toString
([f=Qt.TextDate])def
toString
(format)def
weekNumber
()def
year
()
Static functions¶
def
currentDate
()def
fromJulianDay
(jd_)def
fromString
(s, format)def
fromString
(s[, f=Qt.TextDate])def
isLeapYear
(year)def
isValid
(y, m, d)def
longDayName
(weekday[, type=DateFormat])def
longMonthName
(month[, type=DateFormat])def
shortDayName
(weekday[, type=DateFormat])def
shortMonthName
(month[, type=DateFormat])
Detailed Description¶
A
QDate
object encodes a calendar date, i.e. year, month, and day numbers, in the proleptic Gregorian calendar by default. It can read the current date from the system clock. It provides functions for comparing dates, and for manipulating dates. For example, it is possible to add and subtract days, months, and years to dates.A
QDate
object is typically created by giving the year, month, and day numbers explicitly. Note thatQDate
interprets two digit years as presented, i.e., as years 0 through 99, without adding any offset. AQDate
can also be constructed with the static functioncurrentDate()
, which creates aQDate
object containing the system clock’s date. An explicit date can also be set usingsetDate()
. ThefromString()
function returns aQDate
given a string and a date format which is used to interpret the date within the string.The
year()
,month()
, andday()
functions provide access to the year, month, and day numbers. Also,dayOfWeek()
anddayOfYear()
functions are provided. The same information is provided in textual format by thetoString()
,shortDayName()
,longDayName()
,shortMonthName()
, andlongMonthName()
functions.
QDate
provides a full set of operators to compare twoQDate
objects where smaller means earlier, and larger means later.You can increment (or decrement) a date by a given number of days using
addDays()
. Similarly you can useaddMonths()
andaddYears()
. ThedaysTo()
function returns the number of days between two dates.The
daysInMonth()
anddaysInYear()
functions return how many days there are in this date’s month and year, respectively. TheisLeapYear()
function indicates whether a date is in a leap year.
Remarks¶
No Year 0¶
Range of Valid Dates¶
Dates are stored internally as a Julian Day number, an integer count of every day in a contiguous range, with 24 November 4714 BCE in the Gregorian calendar being Julian Day 0 (1 January 4713 BCE in the Julian calendar). As well as being an efficient and accurate way of storing an absolute date, it is suitable for converting a Date into other calendar systems such as Hebrew, Islamic or Chinese. The Julian Day number can be obtained using
toJulianDay()
and can be set usingfromJulianDay()
.The range of dates able to be stored by
QDate
as a Julian Day number is for technical reasons limited to between -784350574879 and 784354017364, which means from before 2 billion BCE to after 2 billion CE.See also
-
class
QDate
¶ QDate(QDate)
QDate(y, m, d)
- param y
int
- param m
int
- param QDate
- param d
int
Constructs a null date. Null dates are invalid.
Constructs a date with year
y
, monthm
and dayd
.If the specified date is invalid, the date is not set and
isValid()
returnsfalse
.Warning
Years 1 to 99 are interpreted as is. Year 0 is invalid.
See also
-
PySide2.QtCore.QDate.
MonthNameType
¶ This enum describes the types of the string representation used for the month name.
Constant
Description
QDate.DateFormat
This type of name can be used for date-to-string formatting.
QDate.StandaloneFormat
This type is used when you need to enumerate months or weekdays. Usually standalone names are represented in singular forms with capitalized first letter.
-
PySide2.QtCore.QDate.
__reduce__
()¶ - Return type
PyObject
-
PySide2.QtCore.QDate.
__repr__
()¶ - Return type
PyObject
-
PySide2.QtCore.QDate.
addDays
(days)¶ - Parameters
days –
qint64
- Return type
Returns a
QDate
object containing a datendays
later than the date of this object (or earlier ifndays
is negative).Returns a null date if the current date is invalid or the new date is out of range.
See also
-
PySide2.QtCore.QDate.
addMonths
(months)¶ - Parameters
months –
int
- Return type
Returns a
QDate
object containing a datenmonths
later than the date of this object (or earlier ifnmonths
is negative).Note
If the ending day/month combination does not exist in the resulting month/year, this function will return a date that is the latest valid date.
See also
-
PySide2.QtCore.QDate.
addYears
(years)¶ - Parameters
years –
int
- Return type
Returns a
QDate
object containing a datenyears
later than the date of this object (or earlier ifnyears
is negative).Note
If the ending day/month combination does not exist in the resulting year (i.e., if the date was Feb 29 and the final year is not a leap year), this function will return a date that is the latest valid date (that is, Feb 28).
See also
-
static
PySide2.QtCore.QDate.
currentDate
()¶ - Return type
Returns the current date, as reported by the system clock.
See also
-
PySide2.QtCore.QDate.
day
()¶ - Return type
int
Returns the day of the month (1 to 31) of this date.
Returns 0 if the date is invalid.
See also
-
PySide2.QtCore.QDate.
dayOfWeek
()¶ - Return type
int
Returns the weekday (1 = Monday to 7 = Sunday) for this date.
Returns 0 if the date is invalid.
See also
day()
dayOfYear()
DayOfWeek
-
PySide2.QtCore.QDate.
dayOfYear
()¶ - Return type
int
Returns the day of the year (1 to 365 or 366 on leap years) for this date.
Returns 0 if the date is invalid.
See also
-
PySide2.QtCore.QDate.
daysInMonth
()¶ - Return type
int
Returns the number of days in the month (28 to 31) for this date.
Returns 0 if the date is invalid.
See also
-
PySide2.QtCore.QDate.
daysInYear
()¶ - Return type
int
Returns the number of days in the year (365 or 366) for this date.
Returns 0 if the date is invalid.
See also
-
PySide2.QtCore.QDate.
daysTo
(arg__1)¶ - Parameters
arg__1 –
QDate
- Return type
qint64
Returns the number of days from this date to
d
(which is negative ifd
is earlier than this date).Returns 0 if either date is invalid.
Example:
d1 = QDate(1995, 5, 17) # May 17, 1995 d2 = QDate(1995, 5, 20) # May 20, 1995 d1.daysTo(d2) # returns 3 d2.daysTo(d1) # returns -3
See also
-
static
PySide2.QtCore.QDate.
fromJulianDay
(jd_)¶ - Parameters
jd –
qint64
- Return type
Converts the Julian day
jd
to aQDate
.See also
-
static
PySide2.QtCore.QDate.
fromString
(s[, f=Qt.TextDate])¶ - Parameters
s – unicode
f –
DateFormat
- Return type
-
static
PySide2.QtCore.QDate.
fromString
(s, format) - Parameters
s – unicode
format – unicode
- Return type
-
PySide2.QtCore.QDate.
getDate
(year, month, day)¶ - Parameters
year –
int
month –
int
day –
int
Extracts the date’s year, month, and day, and assigns them to *``year`` , *``month`` , and *``day`` . The pointers may be null.
Returns 0 if the date is invalid.
Note
In Qt versions prior to 5.7, this function is marked as non-
const
.
-
static
PySide2.QtCore.QDate.
isLeapYear
(year)¶ - Parameters
year –
int
- Return type
bool
Returns
true
if the specifiedyear
is a leap year; otherwise returnsfalse
.
-
PySide2.QtCore.QDate.
isNull
()¶ - Return type
bool
Returns
true
if the date is null; otherwise returnsfalse
. A null date is invalid.Note
The behavior of this function is equivalent to
isValid()
.See also
-
PySide2.QtCore.QDate.
isValid
()¶ - Return type
bool
Returns
true
if this date is valid; otherwise returnsfalse
.See also
-
static
PySide2.QtCore.QDate.
isValid
(y, m, d) - Parameters
y –
int
m –
int
d –
int
- Return type
bool
This is an overloaded function.
Returns
true
if the specified date (year
,month
, andday
) is valid; otherwise returnsfalse
.Example:
QDate.isValid(2002, 5, 17) # True QDate.isValid(2002, 2, 30) # False (Feb 30 does not exist) QDate.isValid(2004, 2, 29) # True (2004 is a leap year) QDate.isValid(2000, 2, 29) # True (2000 is a leap year) QDate.isValid(2006, 2, 29) # False (2006 is not a leap year) QDate.isValid(2100, 2, 29) # False (2100 is not a leap year) QDate.isValid(1202, 6, 6) # True (even though 1202 is pre-Gregorian)
-
static
PySide2.QtCore.QDate.
longDayName
(weekday[, type=DateFormat])¶ - Parameters
weekday –
int
type –
MonthNameType
- Return type
unicode
Returns the long name of the
weekday
for the representation specified bytype
.The days are enumerated using the following convention:
1 = “Monday”
2 = “Tuesday”
3 = “Wednesday”
4 = “Thursday”
5 = “Friday”
6 = “Saturday”
7 = “Sunday”
The day names will be localized according to the system’s locale settings, i.e. using
system()
.Returns an empty string if the date is invalid.
-
static
PySide2.QtCore.QDate.
longMonthName
(month[, type=DateFormat])¶ - Parameters
month –
int
type –
MonthNameType
- Return type
unicode
Returns the long name of the
month
for the representation specified bytype
.The months are enumerated using the following convention:
1 = “January”
2 = “February”
3 = “March”
4 = “April”
5 = “May”
6 = “June”
7 = “July”
8 = “August”
9 = “September”
10 = “October”
11 = “November”
12 = “December”
The month names will be localized according to the system’s locale settings, i.e. using
system()
.Returns an empty string if the date is invalid.
-
PySide2.QtCore.QDate.
month
()¶ - Return type
int
Returns the number corresponding to the month of this date, using the following convention:
1 = “January”
2 = “February”
3 = “March”
4 = “April”
5 = “May”
6 = “June”
7 = “July”
8 = “August”
9 = “September”
10 = “October”
11 = “November”
12 = “December”
Returns 0 if the date is invalid.
-
PySide2.QtCore.QDate.
__ne__
(other)¶ - Parameters
other –
QDate
- Return type
bool
Returns
true
if this date is different fromd
; otherwise returnsfalse
.
-
PySide2.QtCore.QDate.
__eq__
(other)¶ - Parameters
other –
QDate
- Return type
bool
Returns
true
if this date is equal tod
; otherwise returns false.
-
PySide2.QtCore.QDate.
__gt__
(other)¶ - Parameters
other –
QDate
- Return type
bool
Returns
true
if this date is later thand
; otherwise returns false.
-
PySide2.QtCore.QDate.
__ge__
(other)¶ - Parameters
other –
QDate
- Return type
bool
Returns
true
if this date is later than or equal tod
; otherwise returnsfalse
.
-
PySide2.QtCore.QDate.
setDate
(year, month, day)¶ - Parameters
year –
int
month –
int
day –
int
- Return type
bool
Sets the date’s
year
,month
, andday
. Returnstrue
if the date is valid; otherwise returnsfalse
.If the specified date is invalid, the
QDate
object is set to be invalid.See also
-
static
PySide2.QtCore.QDate.
shortDayName
(weekday[, type=DateFormat])¶ - Parameters
weekday –
int
type –
MonthNameType
- Return type
unicode
Returns the short name of the
weekday
for the representation specified bytype
.The days are enumerated using the following convention:
1 = “Mon”
2 = “Tue”
3 = “Wed”
4 = “Thu”
5 = “Fri”
6 = “Sat”
7 = “Sun”
The day names will be localized according to the system’s locale settings, i.e. using
system()
.Returns an empty string if the date is invalid.
-
static
PySide2.QtCore.QDate.
shortMonthName
(month[, type=DateFormat])¶ - Parameters
month –
int
type –
MonthNameType
- Return type
unicode
Returns the short name of the
month
for the representation specified bytype
.The months are enumerated using the following convention:
1 = “Jan”
2 = “Feb”
3 = “Mar”
4 = “Apr”
5 = “May”
6 = “Jun”
7 = “Jul”
8 = “Aug”
9 = “Sep”
10 = “Oct”
11 = “Nov”
12 = “Dec”
The month names will be localized according to the system’s locale settings, i.e. using
system()
.Returns an empty string if the date is invalid.
-
PySide2.QtCore.QDate.
toJulianDay
()¶ - Return type
qint64
Converts the date to a Julian day.
See also
-
PySide2.QtCore.QDate.
toPython
()¶ - Return type
PyObject
-
PySide2.QtCore.QDate.
toString
([f=Qt.TextDate])¶ - Parameters
f –
DateFormat
- Return type
unicode
This is an overloaded function.
Returns the date as a string. The
format
parameter determines the format of the string.If the
format
isTextDate
, the string is formatted in the default way.shortDayName()
andshortMonthName()
are used to generate the string, so the day and month names will be localized names using the system locale, i.e.system()
. An example of this formatting is “Sat May 20 1995”.If the
format
isISODate
, the string format corresponds to the ISO 8601 extended specification for representations of dates and times, taking the form yyyy-MM-dd, where yyyy is the year, MM is the month of the year (between 01 and 12), and dd is the day of the month between 01 and 31.If the
format
isSystemLocaleShortDate
orSystemLocaleLongDate
, the string format depends on the locale settings of the system. Identical to callingsystem()
.toString
(date,ShortFormat
) orsystem()
.toString
(date,LongFormat
).If the
format
isDefaultLocaleShortDate
orDefaultLocaleLongDate
, the string format depends on the default application locale. This is the locale set withsetDefault()
, or the system locale if no default locale has been set. Identical to callingShortFormat)
orLongFormat)
.If the
format
isRFC2822Date
, the string is formatted in an RFC 2822 compatible way. An example of this formatting is “20 May 1995”.If the date is invalid, an empty string will be returned.
Warning
The
ISODate
format is only valid for years in the range 0 to 9999. This restriction may apply to locale-aware formats as well, depending on the locale settings.
-
PySide2.QtCore.QDate.
toString
(format) - Parameters
format – unicode
- Return type
unicode
-
PySide2.QtCore.QDate.
weekNumber
()¶ - Return type
(week, yearNumber)
Returns the week number (1 to 53), and stores the year in *``yearNumber`` unless
yearNumber
is null (the default).Returns 0 if the date is invalid.
In accordance with ISO 8601, weeks start on Monday and the first Thursday of a year is always in week 1 of that year. Most years have 52 weeks, but some have 53.
*``yearNumber`` is not always the same as
year()
. For example, 1 January 2000 has week number 52 in the year 1999, and 31 December 2002 has week number 1 in the year 2003.See also
-
PySide2.QtCore.QDate.
year
()¶ - Return type
int
Returns the year of this date. Negative numbers indicate years before 1 CE, such that year -44 is 44 BCE.
Returns 0 if the date is invalid.
© 2018 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.