String List Model Example¶
A model may be a simple ‘list’, which provides the contents of the list via the modelData role.
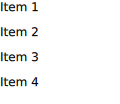
from pathlib import Path
import sys
from PySide6.QtCore import QUrl
from PySide6.QtGui import QGuiApplication
from PySide6.QtQuick import QQuickView
# This example illustrates exposing a QStringList as a model in QML
if __name__ == '__main__':
app = QGuiApplication(sys.argv)
dataList = ["Item 1", "Item 2", "Item 3", "Item 4"]
view = QQuickView()
view.setInitialProperties({"model": dataList })
qml_file = Path(__file__).parent / "view.qml"
view.setSource(QUrl.fromLocalFile(qml_file))
view.show()
r = app.exec()
del view
sys.exit(r)
import QtQuick
ListView {
width: 100
height: 100
required model
delegate: Rectangle {
required property string modelData
height: 25
width: 100
Text { text: parent.modelData }
}
}
© 2022 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.