Introduction Example Qt Quick 3D¶
This example gives an introductory overview of the basic Quick 3D features by going through the code of a simple example.
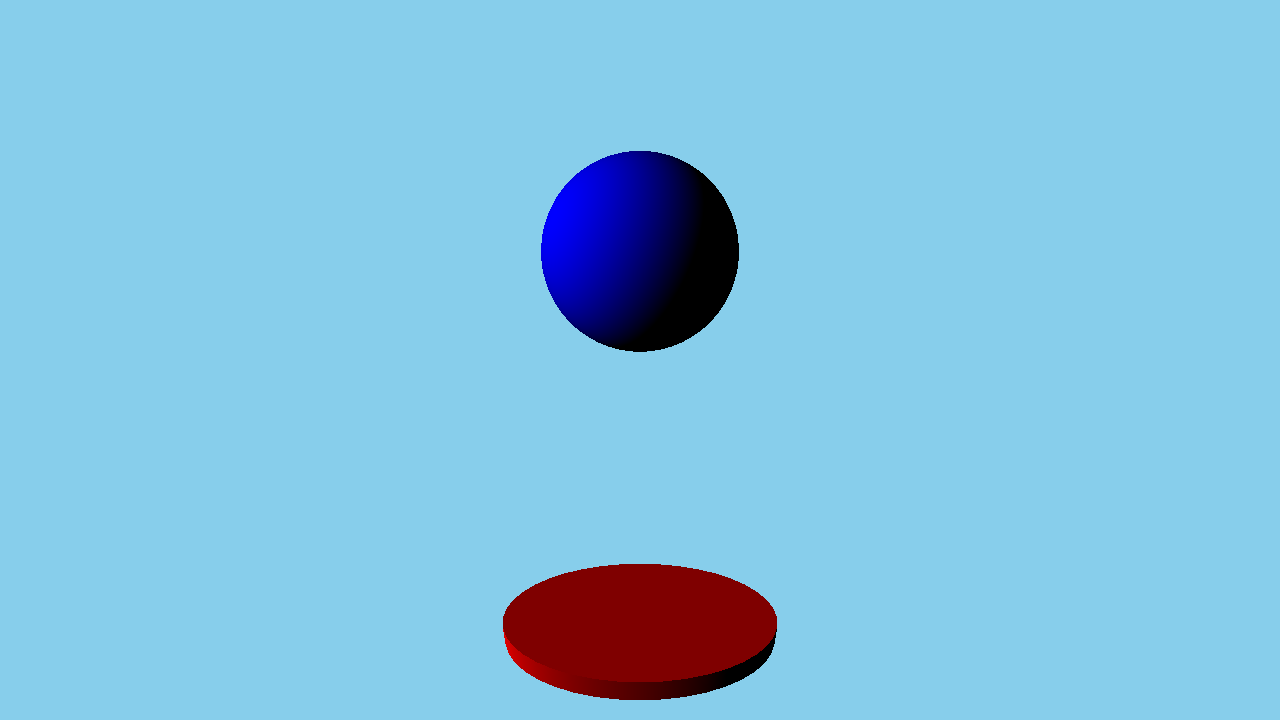
import os
import sys
from pathlib import Path
from PySide6.QtCore import QUrl
from PySide6.QtGui import QGuiApplication, QSurfaceFormat
from PySide6.QtQml import QQmlApplicationEngine
from PySide6.QtQuick3D import QQuick3D
if __name__ == "__main__":
app = QGuiApplication(sys.argv)
QSurfaceFormat.setDefaultFormat(QQuick3D.idealSurfaceFormat(4))
engine = QQmlApplicationEngine()
qml_file = os.fspath(Path(__file__).resolve().parent / 'main.qml')
engine.load(QUrl.fromLocalFile(qml_file))
if not engine.rootObjects():
sys.exit(-1)
sys.exit(app.exec())
//! [import]
import QtQuick
import QtQuick3D
//! [import]
Window {
id: window
width: 1280
height: 720
visible: true
View3D {
id: view
anchors.fill: parent
//! [environment]
environment: SceneEnvironment {
clearColor: "skyblue"
backgroundMode: SceneEnvironment.Color
}
//! [environment]
//! [camera]
PerspectiveCamera {
position: Qt.vector3d(0, 200, 300)
eulerRotation.x: -30
}
//! [camera]
//! [light]
DirectionalLight {
eulerRotation.x: -30
eulerRotation.y: -70
}
//! [light]
//! [objects]
Model {
position: Qt.vector3d(0, -200, 0)
source: "#Cylinder"
scale: Qt.vector3d(2, 0.2, 1)
materials: [ DefaultMaterial {
diffuseColor: "red"
}
]
}
Model {
position: Qt.vector3d(0, 150, 0)
source: "#Sphere"
materials: [ DefaultMaterial {
diffuseColor: "blue"
}
]
//! [animation]
SequentialAnimation on y {
loops: Animation.Infinite
NumberAnimation {
duration: 3000
to: -150
from: 150
easing.type:Easing.InQuad
}
NumberAnimation {
duration: 3000
to: 150
from: -150
easing.type:Easing.OutQuad
}
}
//! [animation]
}
//! [objects]
}
}
© 2022 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.