Warning
This section contains snippets that were automatically translated from C++ to Python and may contain errors.
Bluetooth Low Energy Scanner#
An application designed to browse the content of Bluetooth Low Energy peripheral devices. The example demonstrates the use of all Qt Bluetooth Low Energy classes.
The Bluetooth Low Energy Scanner Example shows how to develop Bluetooth Low Energy applications using the Qt Bluetooth API . The application covers scanning for Low Energy devices, scanning their services and reading the service characteristics and descriptors.
The example introduces the following Qt classes:
The example can be used with any arbitrary Bluetooth Low Energy peripheral device. It creates a snapshot of all services, characteristics and descriptors and presents them to the user. Therefore the application provides an easy way of browsing the content offered by a peripheral device.
Running the Example#
To run the example from Qt Creator, open the Welcome mode and select the example from Examples. For more information, visit Building and Running an Example.
Requesting Permission to use Bluetooth#
On certain platforms, it is required to explicitly grant permissions for using Bluetooth. The example uses BluetoothPermission
QML object to check and request the permissions, if required:
BluetoothPermission { id: permission communicationModes: BluetoothPermission.Access onStatusChanged: { if (permission.status === Qt.PermissionStatus.Denied) Device.update = "Bluetooth permission required" else if (permission.status === Qt.PermissionStatus.Granted) devicesPage.toggleDiscovery() } }
The permission request dialog is triggered when the user tries to start the device discovery, and the permission status is Undetermined
:
onButtonClick: { if (permission.status === Qt.PermissionStatus.Undetermined) permission.request() else if (permission.status === Qt.PermissionStatus.Granted) devicesPage.toggleDiscovery() }
The device discovery starts if the permission is granted by the user. Otherwise the application is non-functional.
Scanning for Devices#
The first step is to find all peripheral devices. The devices can be found using the QBluetoothDeviceDiscoveryAgent
class. The discovery process is started using start()
. Each new device is advertised via the deviceDiscovered()
signal:
discoveryAgent = QBluetoothDeviceDiscoveryAgent(self) discoveryAgent.setLowEnergyDiscoveryTimeout(25000) discoveryAgent.deviceDiscovered.connect( self.addDevice) discoveryAgent.errorOccurred.connect( self.deviceScanError) discoveryAgent.finished.connect( self.deviceScanFinished) discoveryAgent.canceled.connect( self.deviceScanFinished) discoveryAgent.start(QBluetoothDeviceDiscoveryAgent.LowEnergyMethod)
The below addDevice()
slot is triggered as a reaction to the discovery of a new device. It filters all found devices which have the LowEnergyCoreConfiguration
flag and adds them to a list which is shown to the user. The deviceDiscovered()
signal may be emitted multiple times for the same device as more details are discovered. Here we match these device discoveries so that the user only sees the individual devices:
def addDevice(self, info): if info.coreConfigurations() QBluetoothDeviceInfo.LowEnergyCoreConfiguration: devInfo = DeviceInfo(info) it = std::find_if(devices.begin(), devices.end(), [devInfo](DeviceInfo dev) { return devInfo.getAddress() == dev.getAddress() }) if it == devices.end(): devices.append(devInfo) else: oldDev = it it = devInfo del oldDev devicesUpdated.emit()
The list of devices may look like in the image below.
Note
It is a prerequisite that the remote devices actively advertise their presence.
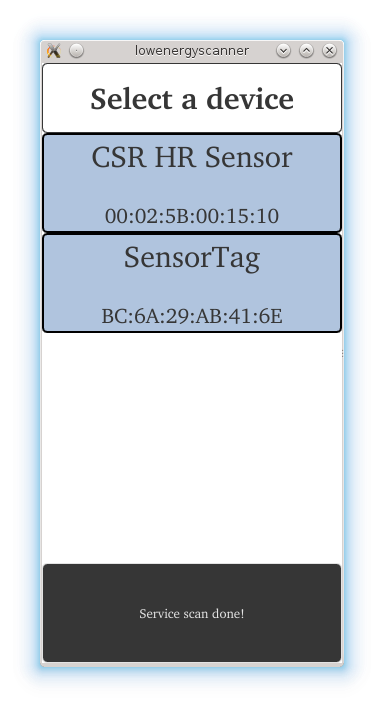
Connecting to Services#
After the user has selected a device from the list the application connects to the device and scans all services. The QLowEnergyController
class is used to connect to the device. The connectToDevice()
function triggers the connection process which lasts until the connected()
signal is received or an error has occurred:
if not controller: # Connecting signals and slots for connecting to LE services. controller = QLowEnergyController.createCentral(currentDevice.getDevice(), self) controller.connected.connect( self.deviceConnected) controller.errorOccurred.connect(self.errorReceived) controller.disconnected.connect( self.deviceDisconnected) controller.serviceDiscovered.connect( self.addLowEnergyService) controller.discoveryFinished.connect( self.serviceScanDone) if isRandomAddress(): controller.setRemoteAddressType(QLowEnergyController.RandomAddress) else: controller.setRemoteAddressType(QLowEnergyController.PublicAddress) controller.connectToDevice()
The slot triggered by the connected()
signal immediately calls discoverServices()
to start the service discovery on the connected peripheral device.
controller.discoverServices()
The resulting list is presented to the user.The image below displays the results when the SensorTag device is selected. The view lists the names of the services, whether they are primary or secondary services and the UUID which determines the service type.
As soon as the service is chosen the related QLowEnergyService
instance is created to permit interaction with it:
service = controller.createServiceObject(serviceUuid) if not service: qWarning() << "Cannot create service for uuid" return
The service object provides the required signals and functions to discover the service details, read and write characteristics and descriptors, as well as receive data change notifications. Change notifications can be triggered as a result of writing a value or due to an on-device update potentially triggered by the internal logic. During the initial detail search the service’s state()
transitions from RemoteService
to RemoteServiceDiscovering
and eventually ends with RemoteServiceDiscovered
:
service.stateChanged.connect( self.serviceDetailsDiscovered) service.discoverDetails() setUpdate("Back\n(Discovering details...)")
Reading Service Data#
Upon selection of a service the service details are shown. Each characteristic is listed together with its name, UUID, value, handle and properties.
It is possible to retrieve the service’s characteristics via characteristics()
and in turn, each descriptor can be obtained via descriptors()
.
chars = service.characteristics() for ch in chars: cInfo = CharacteristicInfo(ch) m_characteristics.append(cInfo)
Although the example application does not display descriptors it uses descriptors to get the name of an individual characteristic if its name cannot be discerned based on its UUID. The second way to obtain the name is the existence of a descriptor of the type CharacteristicUserDescription
. The code below demonstrates how this may be achieved:
name = m_characteristic.name() if not name.isEmpty(): return name # find descriptor with CharacteristicUserDescription descriptors = m_characteristic.descriptors() for descriptor in descriptors: if descriptor.type() == QBluetoothUuid.DescriptorType.CharacteristicUserDescription: name = descriptor.value() break