Chapter 3 - Create an empty QMainWindow¶
You can now think of presenting your data in a UI. A QMainWindow provides a convenient structure for GUI applications, such as a menu bar and status bar. The following image shows the layout that QMainWindow offers out-of-the box:
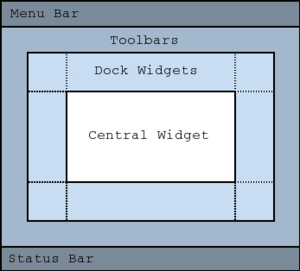
In this case, let your application inherit from QMainWindow, and add the following UI elements:
A “File” menu to open a File dialog.
An “Exit” menu close the window.
A status message on the status bar when the application starts.
In addition, you can define a fixed size for the window or adjust it based on the resolution you currently have. In the following snippet, you will see how window size is defined based on available screen width (80%) and height (70%).
Note
You can achieve a similar structure using other Qt elements like QMenuBar, QWidget, and QStatusBar. Refer the QMainWindow layout for guidance.
1
2from PySide6.QtCore import Slot
3from PySide6.QtGui import QAction, QKeySequence
4from PySide6.QtWidgets import QMainWindow
5
6
7class MainWindow(QMainWindow):
8 def __init__(self):
9 QMainWindow.__init__(self)
10 self.setWindowTitle("Eartquakes information")
11
12 # Menu
13 self.menu = self.menuBar()
14 self.file_menu = self.menu.addMenu("File")
15
16 # Exit QAction
17 exit_action = QAction("Exit", self)
18 exit_action.setShortcut(QKeySequence.Quit)
19 exit_action.triggered.connect(self.close)
20
21 self.file_menu.addAction(exit_action)
22
23 # Status Bar
24 self.status = self.statusBar()
25 self.status.showMessage("Data loaded and plotted")
26
27 # Window dimensions
28 geometry = self.screen().availableGeometry()
29 self.setFixedSize(geometry.width() * 0.8, geometry.height() * 0.7)
Try running the script to see what output you get with it.