QDate¶
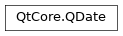
Synopsis¶
Functions¶
def
__eq__
(other)def
__ge__
(other)def
__gt__
(other)def
__le__
(other)def
__lt__
(other)def
__ne__
(other)def
__reduce__
()def
__repr__
()def
addDays
(days)def
addMonths
(months)def
addMonths
(months, cal)def
addYears
(years)def
addYears
(years, cal)def
day
()def
day
(cal)def
dayOfWeek
()def
dayOfWeek
(cal)def
dayOfYear
()def
dayOfYear
(cal)def
daysInMonth
()def
daysInMonth
(cal)def
daysInYear
()def
daysInYear
(cal)def
daysTo
(arg__1)def
endOfDay
([spec=Qt.LocalTime[, offsetSeconds=0]])def
endOfDay
(zone)def
getDate
(year, month, day)def
isNull
()def
isValid
()def
month
()def
month
(cal)def
setDate
(year, month, day)def
setDate
(year, month, day, cal)def
startOfDay
([spec=Qt.LocalTime[, offsetSeconds=0]])def
startOfDay
(zone)def
toJulianDay
()def
toPython
()def
toString
([format=Qt.TextDate])def
toString
(format)def
toString
(format, cal)def
toString
(format, cal)def
weekNumber
()def
year
()def
year
(cal)
Static functions¶
def
currentDate
()def
fromJulianDay
(jd_)def
fromString
(s, format)def
fromString
(s, format, cal)def
fromString
(s[, f=Qt.TextDate])def
isLeapYear
(year)def
isValid
(y, m, d)def
longDayName
(weekday[, type=DateFormat])def
longMonthName
(month[, type=DateFormat])def
shortDayName
(weekday[, type=DateFormat])def
shortMonthName
(month[, type=DateFormat])
Detailed Description¶
A
QDate
object represents a particular day, regardless of calendar, locale or other settings used when creating it or supplied by the system. It can report the year, month and day of the month that represent the day with respect to the proleptic Gregorian calendar or any calendar supplied as aQCalendar
object.A
QDate
object is typically created by giving the year, month, and day numbers explicitly. Note thatQDate
interprets year numbers less than 100 as presented, i.e., as years 1 through 99, without adding any offset. The static functioncurrentDate()
creates aQDate
object containing the date read from the system clock. An explicit date can also be set usingsetDate()
. ThefromString()
function returns aQDate
given a string and a date format which is used to interpret the date within the string.The
year()
,month()
, andday()
functions provide access to the year, month, and day numbers. When more than one of these values is needed, it is more efficient to callpartsFromDate()
, to save repeating (potentially expensive) calendrical calculations.Also,
dayOfWeek()
anddayOfYear()
functions are provided. The same information is provided in textual format bytoString()
.QLocale
can map the day numbers to names,QCalendar
can map month numbers to names.
QDate
provides a full set of operators to compare twoQDate
objects where smaller means earlier, and larger means later.You can increment (or decrement) a date by a given number of days using
addDays()
. Similarly you can useaddMonths()
andaddYears()
. ThedaysTo()
function returns the number of days between two dates.The
daysInMonth()
anddaysInYear()
functions return how many days there are in this date’s month and year, respectively. TheisLeapYear()
function indicates whether a date is in a leap year.QCalendar
can also supply this information, in some cases more conveniently.
Remarks¶
No Year 0¶
In the Gregorian calendar, there is no year 0. Dates in that year are considered invalid. The year -1 is the year “1 before Christ” or “1 before common era.” The day before 1 January 1 CE,
QDate
(1, 1, 1), is 31 December 1 BCE,QDate
(-1, 12, 31). Various other calendars behave similarly; seehasYearZero()
.
Range of Valid Dates¶
Dates are stored internally as a Julian Day number, an integer count of every day in a contiguous range, with 24 November 4714 BCE in the Gregorian calendar being Julian Day 0 (1 January 4713 BCE in the Julian calendar). As well as being an efficient and accurate way of storing an absolute date, it is suitable for converting a date into other calendar systems such as Hebrew, Islamic or Chinese. The Julian Day number can be obtained using
toJulianDay()
and can be set usingfromJulianDay()
.The range of Julian Day numbers that
QDate
can represent is, for technical reasons, limited to between -784350574879 and 784354017364, which means from before 2 billion BCE to after 2 billion CE. This is more than seven times as wide as the range of dates aQDateTime
can represent.
- class PySide2.QtCore.QDate¶
PySide2.QtCore.QDate(QDate)
PySide2.QtCore.QDate(y, m, d)
PySide2.QtCore.QDate(y, m, d, cal)
- param y:
int
- param m:
int
- param cal:
- param QDate:
- param d:
int
Constructs a null date. Null dates are invalid.
Constructs a date with year
y
, monthm
and dayd
.The date is understood in terms of the Gregorian calendar. If the specified date is invalid, the date is not set and
isValid()
returnsfalse
.Warning
Years 1 to 99 are interpreted as is. Year 0 is invalid.
See also
- PySide2.QtCore.QDate.MonthNameType¶
This enum describes the types of the string representation used for the month name.
Constant
Description
QDate.DateFormat
This type of name can be used for date-to-string formatting.
QDate.StandaloneFormat
This type is used when you need to enumerate months or weekdays. Usually standalone names are represented in singular forms with capitalized first letter.
- PySide2.QtCore.QDate.__reduce__()¶
- Return type:
object
- PySide2.QtCore.QDate.__repr__()¶
- Return type:
object
- PySide2.QtCore.QDate.addDays(days)¶
- Parameters:
days – int
- Return type:
Returns a
QDate
object containing a datendays
later than the date of this object (or earlier ifndays
is negative).Returns a null date if the current date is invalid or the new date is out of range.
See also
- PySide2.QtCore.QDate.addMonths(months)¶
- Parameters:
months – int
- Return type:
This is an overloaded function.
- PySide2.QtCore.QDate.addMonths(months, cal)
- Parameters:
months – int
cal –
PySide2.QtCore.QCalendar
- Return type:
Returns a
QDate
object containing a datenmonths
later than the date of this object (or earlier ifnmonths
is negative).Uses
cal
as calendar, if supplied, else the Gregorian calendar.Note
If the ending day/month combination does not exist in the resulting month/year, this function will return a date that is the latest valid date in the selected month.
See also
- PySide2.QtCore.QDate.addYears(years)¶
- Parameters:
years – int
- Return type:
This is an overloaded function.
- PySide2.QtCore.QDate.addYears(years, cal)
- Parameters:
years – int
cal –
PySide2.QtCore.QCalendar
- Return type:
Returns a
QDate
object containing a datenyears
later than the date of this object (or earlier ifnyears
is negative).Uses
cal
as calendar, if supplied, else the Gregorian calendar.Note
If the ending day/month combination does not exist in the resulting year (e.g., for the Gregorian calendar, if the date was Feb 29 and the final year is not a leap year), this function will return a date that is the latest valid date in the given month (in the example, Feb 28).
See also
- static PySide2.QtCore.QDate.currentDate()¶
- Return type:
Returns the current date, as reported by the system clock.
See also
- PySide2.QtCore.QDate.day()¶
- Return type:
int
This is an overloaded function.
- PySide2.QtCore.QDate.day(cal)
- Parameters:
cal –
PySide2.QtCore.QCalendar
- Return type:
int
Returns the day of the month for this date.
Uses
cal
as calendar if supplied, else the Gregorian calendar (for which the return ranges from 1 to 31). Returns 0 if the date is invalid.See also
- PySide2.QtCore.QDate.dayOfWeek()¶
- Return type:
int
This is an overloaded function.
- PySide2.QtCore.QDate.dayOfWeek(cal)
- Parameters:
cal –
PySide2.QtCore.QCalendar
- Return type:
int
Returns the weekday (1 = Monday to 7 = Sunday) for this date.
Uses
cal
as calendar if supplied, else the Gregorian calendar. Returns 0 if the date is invalid. Some calendars may give special meaning (e.g. intercallary days) to values greater than 7.See also
day()
dayOfYear()
dayOfWeek()
DayOfWeek
- PySide2.QtCore.QDate.dayOfYear(cal)¶
- Parameters:
cal –
PySide2.QtCore.QCalendar
- Return type:
int
Returns the day of the year (1 for the first day) for this date.
Uses
cal
as calendar if supplied, else the Gregorian calendar. Returns 0 if either the date or the first day of its year is invalid.See also
- PySide2.QtCore.QDate.dayOfYear()
- Return type:
int
This is an overloaded function.
- PySide2.QtCore.QDate.daysInMonth()¶
- Return type:
int
This is an overloaded function.
- PySide2.QtCore.QDate.daysInMonth(cal)
- Parameters:
cal –
PySide2.QtCore.QCalendar
- Return type:
int
Returns the number of days in the month for this date.
Uses
cal
as calendar if supplied, else the Gregorian calendar (for which the result ranges from 28 to 31). Returns 0 if the date is invalid.
- PySide2.QtCore.QDate.daysInYear()¶
- Return type:
int
This is an overloaded function.
- PySide2.QtCore.QDate.daysInYear(cal)
- Parameters:
cal –
PySide2.QtCore.QCalendar
- Return type:
int
Returns the number of days in the year for this date.
Uses
cal
as calendar if supplied, else the Gregorian calendar (for which the result is 365 or 366). Returns 0 if the date is invalid.
- PySide2.QtCore.QDate.daysTo(arg__1)¶
- Parameters:
arg__1 –
PySide2.QtCore.QDate
- Return type:
int
Returns the number of days from this date to
d
(which is negative ifd
is earlier than this date).Returns 0 if either date is invalid.
Example:
d1 = QDate(1995, 5, 17) # May 17, 1995 d2 = QDate(1995, 5, 20) # May 20, 1995 d1.daysTo(d2) # returns 3 d2.daysTo(d1) # returns -3
See also
- PySide2.QtCore.QDate.endOfDay([spec=Qt.LocalTime[, offsetSeconds=0]])¶
- Parameters:
spec –
TimeSpec
offsetSeconds – int
- Return type:
- PySide2.QtCore.QDate.endOfDay(zone)
- Parameters:
zone –
PySide2.QtCore.QTimeZone
- Return type:
This is an overloaded function.
- static PySide2.QtCore.QDate.fromJulianDay(jd_)¶
- Parameters:
jd – int
- Return type:
Converts the Julian day
jd
to aQDate
.See also
- static PySide2.QtCore.QDate.fromString(s[, f=Qt.TextDate])¶
- Parameters:
s – str
f –
DateFormat
- Return type:
- static PySide2.QtCore.QDate.fromString(s, format)
- Parameters:
s – str
format – str
- Return type:
- static PySide2.QtCore.QDate.fromString(s, format, cal)
- Parameters:
s – str
format – str
cal –
PySide2.QtCore.QCalendar
- Return type:
Returns the
QDate
represented by thestring
, using theformat
given, or an invalid date if the string cannot be parsed.Uses
cal
as calendar if supplied, else the Gregorian calendar. Ranges of values in the format descriptions below are for the latter; they may be different for other calendars.These expressions may be used for the format:
Expression
Output
d
The day as a number without a leading zero (1 to 31)
dd
The day as a number with a leading zero (01 to 31)
ddd
The abbreviated localized day name (e.g. ‘Mon’ to ‘Sun’). Uses the system locale to localize the name, i.e.
system()
.dddd
The long localized day name (e.g. ‘Monday’ to ‘Sunday’). Uses the system locale to localize the name, i.e.
system()
.M
The month as a number without a leading zero (1 to 12)
MM
The month as a number with a leading zero (01 to 12)
MMM
The abbreviated localized month name (e.g. ‘Jan’ to ‘Dec’). Uses the system locale to localize the name, i.e.
system()
.MMMM
The long localized month name (e.g. ‘January’ to ‘December’). Uses the system locale to localize the name, i.e.
system()
.yy
The year as a two digit number (00 to 99)
yyyy
The year as a four digit number, possibly plus a leading minus sign for negative years.
Note
Unlike the other version of this function, day and month names must be given in the user’s local language. It is only possible to use the English names if the user’s language is English.
All other input characters will be treated as text. Any non-empty sequence of characters enclosed in single quotes will also be treated (stripped of the quotes) as text and not be interpreted as expressions. For example:
date = QDate.fromString("1MM12car2003", "d'MM'MMcaryyyy") # date is 1 December 2003
If the format is not satisfied, an invalid
QDate
is returned. The expressions that don’t expect leading zeroes (d, M) will be greedy. This means that they will use two digits even if this will put them outside the accepted range of values and leaves too few digits for other sections. For example, the following format string could have meant January 30 but the M will grab two digits, resulting in an invalid date:date = QDate.fromString("130", "Md") # invalid
For any field that is not represented in the format the following defaults are used:
Field
Default value
Year
1900
Month
1
Day
1
The following examples demonstrate the default values:
QDate.fromString("1.30", "M.d") # January 30 1900 QDate.fromString("20000110", "yyyyMMdd") # January 10, 2000 QDate.fromString("20000110", "yyyyMd") # January 10, 2000
Note
If localized month and day names are used, please switch to using
system()
.toDate() asQDate
methods shall change to only recognize English (C locale) names at Qt 6.See also
- PySide2.QtCore.QDate.getDate(year, month, day)¶
- Parameters:
year – int
month – int
day – int
Extracts the date’s year, month, and day, and assigns them to *``year`` , *``month`` , and *``day`` . The pointers may be null.
Returns 0 if the date is invalid.
Note
In Qt versions prior to 5.7, this function is marked as non-
const
.See also
- static PySide2.QtCore.QDate.isLeapYear(year)¶
- Parameters:
year – int
- Return type:
bool
Returns
true
if the specifiedyear
is a leap year in the Gregorian calendar; otherwise returnsfalse
.See also
- PySide2.QtCore.QDate.isNull()¶
- Return type:
bool
Returns
true
if the date is null; otherwise returnsfalse
. A null date is invalid.Note
The behavior of this function is equivalent to
isValid()
.See also
- PySide2.QtCore.QDate.isValid()¶
- Return type:
bool
Returns
true
if this date is valid; otherwise returnsfalse
.See also
- static PySide2.QtCore.QDate.isValid(y, m, d)
- Parameters:
y – int
m – int
d – int
- Return type:
bool
This is an overloaded function.
Returns
true
if the specified date (year
,month
, andday
) is valid in the Gregorian calendar; otherwise returnsfalse
.Example:
QDate.isValid(2002, 5, 17) # True QDate.isValid(2002, 2, 30) # False (Feb 30 does not exist) QDate.isValid(2004, 2, 29) # True (2004 is a leap year) QDate.isValid(2000, 2, 29) # True (2000 is a leap year) QDate.isValid(2006, 2, 29) # False (2006 is not a leap year) QDate.isValid(2100, 2, 29) # False (2100 is not a leap year) QDate.isValid(1202, 6, 6) # True (even though 1202 is pre-Gregorian)
See also
- static PySide2.QtCore.QDate.longDayName(weekday[, type=DateFormat])¶
- Parameters:
weekday – int
type –
MonthNameType
- Return type:
str
Note
This function is deprecated.
Returns the long name of the
weekday
for the representation specified bytype
.The days are enumerated using the following convention:
1 = “Monday”
2 = “Tuesday”
3 = “Wednesday”
4 = “Thursday”
5 = “Friday”
6 = “Saturday”
7 = “Sunday”
The day names will be localized according to the system’s locale settings, i.e. using
system()
.Returns an empty string if the date is invalid.
- static PySide2.QtCore.QDate.longMonthName(month[, type=DateFormat])¶
- Parameters:
month – int
type –
MonthNameType
- Return type:
str
Note
This function is deprecated.
Returns the long name of the
month
for the representation specified bytype
.The months are enumerated using the following convention:
1 = “January”
2 = “February”
3 = “March”
4 = “April”
5 = “May”
6 = “June”
7 = “July”
8 = “August”
9 = “September”
10 = “October”
11 = “November”
12 = “December”
The month names will be localized according to the system’s locale settings, i.e. using
system()
.Returns an empty string if the date is invalid.
- PySide2.QtCore.QDate.month()¶
- Return type:
int
This is an overloaded function.
- PySide2.QtCore.QDate.month(cal)
- Parameters:
cal –
PySide2.QtCore.QCalendar
- Return type:
int
Returns the month-number for the date.
Numbers the months of the year starting with 1 for the first. Uses
cal
as calendar if supplied, else the Gregorian calendar, for which the month numbering is as follows:1 = “January”
2 = “February”
3 = “March”
4 = “April”
5 = “May”
6 = “June”
7 = “July”
8 = “August”
9 = “September”
10 = “October”
11 = “November”
12 = “December”
Returns 0 if the date is invalid. Note that some calendars may have more than 12 months in some years.
See also
- PySide2.QtCore.QDate.__ne__(other)¶
- Parameters:
other –
PySide2.QtCore.QDate
- Return type:
bool
Returns
true
if this date is different fromd
; otherwise returnsfalse
.See also
operator==()
- PySide2.QtCore.QDate.__lt__(other)¶
- Parameters:
other –
PySide2.QtCore.QDate
- Return type:
bool
- PySide2.QtCore.QDate.__le__(other)¶
- Parameters:
other –
PySide2.QtCore.QDate
- Return type:
bool
- PySide2.QtCore.QDate.__eq__(other)¶
- Parameters:
other –
PySide2.QtCore.QDate
- Return type:
bool
Returns
true
if this date andd
represent the same day, otherwisefalse
.
- PySide2.QtCore.QDate.__gt__(other)¶
- Parameters:
other –
PySide2.QtCore.QDate
- Return type:
bool
Returns
true
if this date is later thand
; otherwise returns false.
- PySide2.QtCore.QDate.__ge__(other)¶
- Parameters:
other –
PySide2.QtCore.QDate
- Return type:
bool
Returns
true
if this date is later than or equal tod
; otherwise returnsfalse
.
- PySide2.QtCore.QDate.setDate(year, month, day)¶
- Parameters:
year – int
month – int
day – int
- Return type:
bool
Sets this to represent the date, in the Gregorian calendar, with the given
year
,month
andday
numbers. Returns true if the resulting date is valid, otherwise it sets this to represent an invalid date and returns false.See also
- PySide2.QtCore.QDate.setDate(year, month, day, cal)
- Parameters:
year – int
month – int
day – int
cal –
PySide2.QtCore.QCalendar
- Return type:
bool
Sets this to represent the date, in the given calendar
cal
, with the givenyear
,month
andday
numbers. Returns true if the resulting date is valid, otherwise it sets this to represent an invalid date and returns false.See also
- static PySide2.QtCore.QDate.shortDayName(weekday[, type=DateFormat])¶
- Parameters:
weekday – int
type –
MonthNameType
- Return type:
str
Note
This function is deprecated.
Returns the short name of the
weekday
for the representation specified bytype
.The days are enumerated using the following convention:
1 = “Mon”
2 = “Tue”
3 = “Wed”
4 = “Thu”
5 = “Fri”
6 = “Sat”
7 = “Sun”
The day names will be localized according to the system’s locale settings, i.e. using
system()
.Returns an empty string if the date is invalid.
- static PySide2.QtCore.QDate.shortMonthName(month[, type=DateFormat])¶
- Parameters:
month – int
type –
MonthNameType
- Return type:
str
Note
This function is deprecated.
Returns the short name of the
month
for the representation specified bytype
.The months are enumerated using the following convention:
1 = “Jan”
2 = “Feb”
3 = “Mar”
4 = “Apr”
5 = “May”
6 = “Jun”
7 = “Jul”
8 = “Aug”
9 = “Sep”
10 = “Oct”
11 = “Nov”
12 = “Dec”
The month names will be localized according to the system’s locale settings, i.e. using
system()
.Returns an empty string if the date is invalid.
- PySide2.QtCore.QDate.startOfDay([spec=Qt.LocalTime[, offsetSeconds=0]])¶
- Parameters:
spec –
TimeSpec
offsetSeconds – int
- Return type:
- PySide2.QtCore.QDate.startOfDay(zone)
- Parameters:
zone –
PySide2.QtCore.QTimeZone
- Return type:
This is an overloaded function.
- PySide2.QtCore.QDate.toJulianDay()¶
- Return type:
int
Converts the date to a Julian day.
See also
- PySide2.QtCore.QDate.toPython()¶
- Return type:
object
- PySide2.QtCore.QDate.toString([format=Qt.TextDate])¶
- Parameters:
format –
DateFormat
- Return type:
str
This is an overloaded function.
Returns the date as a string. The
format
parameter determines the format of the string.If the
format
isTextDate
, the string is formatted in the default way. The day and month names will be localized names using the system locale, i.e.system()
. An example of this formatting is “Sat May 20 1995”.If the
format
isISODate
, the string format corresponds to the ISO 8601 extended specification for representations of dates and times, taking the form yyyy-MM-dd, where yyyy is the year, MM is the month of the year (between 01 and 12), and dd is the day of the month between 01 and 31.The
format
optionsSystemLocaleDate
,SystemLocaleShortDate
andSystemLocaleLongDate
shall be removed in Qt 6. Their use should be replaced withShortFormat)
orLongFormat)
.The
format
optionsLocaleDate
,DefaultLocaleShortDate
andDefaultLocaleLongDate
shall be removed in Qt 6. Their use should be replaced withShortFormat)
orLongFormat)
.If the
format
isRFC2822Date
, the string is formatted in an RFC 2822 compatible way. An example of this formatting is “20 May 1995”.If the date is invalid, an empty string will be returned.
Warning
The
ISODate
format is only valid for years in the range 0 to 9999.See also
- PySide2.QtCore.QDate.toString(format, cal)
- Parameters:
format –
DateFormat
cal –
PySide2.QtCore.QCalendar
- Return type:
str
Note
This function is deprecated.
- PySide2.QtCore.QDate.toString(format)
- Parameters:
format – str
- Return type:
str
- PySide2.QtCore.QDate.toString(format, cal)
- Parameters:
format – str
cal –
PySide2.QtCore.QCalendar
- Return type:
str
- PySide2.QtCore.QDate.weekNumber()¶
- Return type:
(week, yearNumber)
Returns the ISO 8601 week number (1 to 53).
Returns 0 if the date is invalid. Otherwise, returns the week number for the date. If
yearNumber
is notNone
(its default), stores the year as *``yearNumber`` .In accordance with ISO 8601, each week falls in the year to which most of its days belong, in the Gregorian calendar. As ISO 8601’s week starts on Monday, this is the year in which the week’s Thursday falls. Most years have 52 weeks, but some have 53.
Note
*``yearNumber`` is not always the same as
year()
. For example, 1 January 2000 has week number 52 in the year 1999, and 31 December 2002 has week number 1 in the year 2003.See also
- PySide2.QtCore.QDate.year()¶
- Return type:
int
This is an overloaded function.
- PySide2.QtCore.QDate.year(cal)
- Parameters:
cal –
PySide2.QtCore.QCalendar
- Return type:
int
Returns the year of this date.
Uses
cal
as calendar, if supplied, else the Gregorian calendar.Returns 0 if the date is invalid. For some calendars, dates before their first year may all be invalid.
If using a calendar which has a year 0, check using
isValid()
if the return is 0. Such calendars use negative year numbers in the obvious way, with year 1 preceded by year 0, in turn preceded by year -1 and so on.Some calendars, despite having no year 0, have a conventional numbering of the years before their first year, counting backwards from 1. For example, in the proleptic Gregorian calendar, successive years before 1 CE (the first year) are identified as 1 BCE, 2 BCE, 3 BCE and so on. For such calendars, negative year numbers are used to indicate these years before year 1, with -1 indicating the year before 1.
© 2022 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.