QJsonArray¶
The
QJsonArray
class encapsulates a JSON array. More…
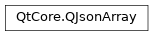
Synopsis¶
Functions¶
def
__add__
(v)def
__eq__
(other)def
__iadd__
(v)def
__lshift__
(v)def
__ne__
(other)def
append
(value)def
at
(i)def
contains
(element)def
count
()def
empty
()def
first
()def
insert
(i, value)def
isEmpty
()def
last
()def
operator[]
(i)def
pop_back
()def
pop_front
()def
prepend
(value)def
push_back
(t)def
push_front
(t)def
removeAt
(i)def
removeFirst
()def
removeLast
()def
replace
(i, value)def
size
()def
swap
(other)def
takeAt
(i)def
toVariantList
()
Static functions¶
def
fromStringList
(list)def
fromVariantList
(list)
Detailed Description¶
A JSON array is a list of values. The list can be manipulated by inserting and removing
QJsonValue
‘s from the array.A
QJsonArray
can be converted to and from aQVariantList
. You can query the number of entries withsize()
,insert()
, andremoveAt()
entries from it and iterate over its content using the standard C++ iterator pattern.
QJsonArray
is an implicitly shared class and shares the data with the document it has been created from as long as it is not being modified.You can convert the array to and from text based JSON through
QJsonDocument
.See also
JSON Support in Qt JSON Save Game Example
- class PySide2.QtCore.QJsonArray¶
PySide2.QtCore.QJsonArray(other)
- param other:
Creates an empty array.
- PySide2.QtCore.QJsonArray.append(value)¶
- Parameters:
value –
PySide2.QtCore.QJsonValue
Inserts
value
at the end of the array.
- PySide2.QtCore.QJsonArray.at(i)¶
- Parameters:
i – int
- Return type:
Returns a
QJsonValue
representing the value for indexi
.The returned
QJsonValue
isUndefined
, ifi
is out of bounds.
- PySide2.QtCore.QJsonArray.contains(element)¶
- Parameters:
element –
PySide2.QtCore.QJsonValue
- Return type:
bool
Returns
true
if the array contains an occurrence ofvalue
, otherwisefalse
.See also
- PySide2.QtCore.QJsonArray.empty()¶
- Return type:
bool
This function is provided for STL compatibility. It is equivalent to
isEmpty()
and returnstrue
if the array is empty.
- PySide2.QtCore.QJsonArray.first()¶
- Return type:
Returns the first value stored in the array.
Same as
at(0)
.See also
- static PySide2.QtCore.QJsonArray.fromStringList(list)¶
- Parameters:
list – list of strings
- Return type:
Converts the string list
list
to aQJsonArray
.The values in
list
will be converted to JSON values.See also
- static PySide2.QtCore.QJsonArray.fromVariantList(list)¶
- Parameters:
list –
- Return type:
Converts the variant list
list
to aQJsonArray
.The
QVariant
values inlist
will be converted to JSON values.Note
Conversion from
QVariant
is not completely lossless. Please see the documentation infromVariant()
for more information.See also
- PySide2.QtCore.QJsonArray.insert(i, value)¶
- Parameters:
i – int
value –
PySide2.QtCore.QJsonValue
- PySide2.QtCore.QJsonArray.isEmpty()¶
- Return type:
bool
Returns
true
if the object is empty. This is the same assize()
== 0.See also
- PySide2.QtCore.QJsonArray.last()¶
- Return type:
Returns the last value stored in the array.
Same as
at(size() - 1)
.See also
- PySide2.QtCore.QJsonArray.__ne__(other)¶
- Parameters:
other –
PySide2.QtCore.QJsonArray
- Return type:
bool
Returns
true
if this array is not equal toother
.
- PySide2.QtCore.QJsonArray.__add__(v)¶
- Parameters:
- Return type:
Returns an array that contains all the items in this array followed by the provided
value
.See also
operator+=()
- PySide2.QtCore.QJsonArray.__iadd__(v)¶
- Parameters:
- Return type:
Appends
value
to the array, and returns a reference to the array itself.See also
append()
operator
- PySide2.QtCore.QJsonArray.__lshift__(v)¶
- Parameters:
- Return type:
- PySide2.QtCore.QJsonArray.__eq__(other)¶
- Parameters:
other –
PySide2.QtCore.QJsonArray
- Return type:
bool
Returns
true
if this array is equal toother
.
- PySide2.QtCore.QJsonArray.operator[](i)
- Parameters:
i – int
- Return type:
This is an overloaded function.
Same as
at()
.
- PySide2.QtCore.QJsonArray.pop_back()¶
This function is provided for STL compatibility. It is equivalent to
removeLast()
. The array must not be empty. If the array can be empty, callisEmpty()
before calling this function.
- PySide2.QtCore.QJsonArray.pop_front()¶
This function is provided for STL compatibility. It is equivalent to
removeFirst()
. The array must not be empty. If the array can be empty, callisEmpty()
before calling this function.
- PySide2.QtCore.QJsonArray.prepend(value)¶
- Parameters:
value –
PySide2.QtCore.QJsonValue
Inserts
value
at the beginning of the array.This is the same as
insert(0, value)
and will prependvalue
to the array.
- PySide2.QtCore.QJsonArray.push_back(t)¶
- Parameters:
This function is provided for STL compatibility. It is equivalent to
append(value)
and will appendvalue
to the array.
- PySide2.QtCore.QJsonArray.push_front(t)¶
- Parameters:
This function is provided for STL compatibility. It is equivalent to
prepend(value)
and will prependvalue
to the array.
- PySide2.QtCore.QJsonArray.removeAt(i)¶
- Parameters:
i – int
Removes the value at index position
i
.i
must be a valid index position in the array (i.e.,0 <= i < size()
).
- PySide2.QtCore.QJsonArray.removeFirst()¶
Removes the first item in the array. Calling this function is equivalent to calling
removeAt(0)
. The array must not be empty. If the array can be empty, callisEmpty()
before calling this function.See also
- PySide2.QtCore.QJsonArray.removeLast()¶
Removes the last item in the array. Calling this function is equivalent to calling
removeAt(size() - 1)
. The array must not be empty. If the array can be empty, callisEmpty()
before calling this function.See also
- PySide2.QtCore.QJsonArray.replace(i, value)¶
- Parameters:
i – int
value –
PySide2.QtCore.QJsonValue
Replaces the item at index position
i
withvalue
.i
must be a valid index position in the array (i.e.,0 <= i < size()
).See also
operator[]()
removeAt()
- PySide2.QtCore.QJsonArray.size()¶
- Return type:
int
Returns the number of values stored in the array.
- PySide2.QtCore.QJsonArray.swap(other)¶
- Parameters:
other –
PySide2.QtCore.QJsonArray
Swaps the array
other
with this. This operation is very fast and never fails.
- PySide2.QtCore.QJsonArray.takeAt(i)¶
- Parameters:
i – int
- Return type:
Removes the item at index position
i
and returns it.i
must be a valid index position in the array (i.e.,0 <= i < size()
).If you don’t use the return value,
removeAt()
is more efficient.See also
- PySide2.QtCore.QJsonArray.toVariantList()¶
- Return type:
Converts this object to a
QVariantList
.Returns the created map.
© 2022 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.