QSizeF¶
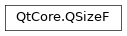
Synopsis¶
Functions¶
def
__add__
(, s2)def
__div__
(, c)def
__eq__
(, s2)def
__iadd__
(arg__1)def
__idiv__
(c)def
__imul__
(c)def
__isub__
(arg__1)def
__mul__
(, c)def
__mul__
(c)def
__ne__
(, s2)def
__reduce__
()def
__repr__
()def
__sub__
(, s2)def
boundedTo
(arg__1)def
expandedTo
(arg__1)def
grownBy
(m)def
height
()def
isEmpty
()def
isNull
()def
isValid
()def
scale
(s, mode)def
scale
(w, h, mode)def
scaled
(s, mode)def
scaled
(w, h, mode)def
setHeight
(h)def
setWidth
(w)def
shrunkBy
(m)def
toSize
()def
toTuple
()def
transpose
()def
transposed
()def
width
()
Detailed Description¶
A size is specified by a
width()
and aheight()
. It can be set in the constructor and changed using thesetWidth()
,setHeight()
, orscale()
functions, or using arithmetic operators. A size can also be manipulated directly by retrieving references to the width and height using therwidth()
andrheight()
functions. Finally, the width and height can be swapped using thetranspose()
function.The
isValid()
function determines if a size is valid. A valid size has both width and height greater than or equal to zero. TheisEmpty()
function returnstrue
if either of the width and height is less than (or equal to) zero, while theisNull()
function returnstrue
only if both the width and the height is zero.Use the
expandedTo()
function to retrieve a size which holds the maximum height and width of this size and a given size. Similarly, theboundedTo()
function returns a size which holds the minimum height and width of this size and a given size.The
QSizeF
class also provides thetoSize()
function returning aQSize
copy of this size, constructed by rounding the width and height to the nearest integers.
QSizeF
objects can be streamed as well as compared.
- class PySide2.QtCore.QSizeF¶
PySide2.QtCore.QSizeF(sz)
PySide2.QtCore.QSizeF(QSizeF)
PySide2.QtCore.QSizeF(w, h)
- param w:
float
- param h:
float
- param QSizeF:
- param sz:
Constructs an invalid size.
See also
Constructs a size with floating point accuracy from the given
size
.See also
Constructs a size with the given
width
andheight
.
- PySide2.QtCore.QSizeF.__reduce__()¶
- Return type:
object
- PySide2.QtCore.QSizeF.__repr__()¶
- Return type:
object
- PySide2.QtCore.QSizeF.boundedTo(arg__1)¶
- Parameters:
arg__1 –
PySide2.QtCore.QSizeF
- Return type:
Returns a size holding the minimum width and height of this size and the given
otherSize
.See also
- PySide2.QtCore.QSizeF.expandedTo(arg__1)¶
- Parameters:
arg__1 –
PySide2.QtCore.QSizeF
- Return type:
Returns a size holding the maximum width and height of this size and the given
otherSize
.See also
- PySide2.QtCore.QSizeF.grownBy(m)¶
- Parameters:
- Return type:
- PySide2.QtCore.QSizeF.height()¶
- Return type:
float
Returns the height.
See also
- PySide2.QtCore.QSizeF.isEmpty()¶
- Return type:
bool
Returns
true
if either of the width and height is less than or equal to 0; otherwise returnsfalse
.
- PySide2.QtCore.QSizeF.isNull()¶
- Return type:
bool
Returns
true
if both the width and height are 0.0 (ignoring the sign); otherwise returnsfalse
.
- PySide2.QtCore.QSizeF.isValid()¶
- Return type:
bool
Returns
true
if both the width and height is equal to or greater than 0; otherwise returnsfalse
.
- PySide2.QtCore.QSizeF.__ne__(s2)¶
- Parameters:
- Return type:
bool
- PySide2.QtCore.QSizeF.__mul__(c)¶
- Parameters:
c – float
- Return type:
- PySide2.QtCore.QSizeF.__mul__(c)
- Parameters:
c – float
- Return type:
- PySide2.QtCore.QSizeF.__imul__(c)¶
- Parameters:
c – float
- Return type:
This is an overloaded function.
Multiplies both the width and height by the given
factor
and returns a reference to the size.See also
- PySide2.QtCore.QSizeF.__add__(s2)¶
- Parameters:
- Return type:
- PySide2.QtCore.QSizeF.__iadd__(arg__1)¶
- Parameters:
arg__1 –
PySide2.QtCore.QSizeF
- Return type:
Adds the given
size
to this size and returns a reference to this size. For example:s = QSizeF( 3, 7) r = QSizeF(-1, 4) s += r # s becomes (2,11)
- PySide2.QtCore.QSizeF.__sub__(s2)¶
- Parameters:
- Return type:
- PySide2.QtCore.QSizeF.__isub__(arg__1)¶
- Parameters:
arg__1 –
PySide2.QtCore.QSizeF
- Return type:
Subtracts the given
size
from this size and returns a reference to this size. For example:s = QSizeF( 3, 7) r = QSizeF(-1, 4) s -= r # s becomes (4,3)
- PySide2.QtCore.QSizeF.__div__(c)¶
- Parameters:
c – float
- Return type:
- PySide2.QtCore.QSizeF.__idiv__(c)¶
- Parameters:
c – float
- Return type:
This is an overloaded function.
Divides both the width and height by the given
divisor
and returns a reference to the size.See also
- PySide2.QtCore.QSizeF.__eq__(s2)¶
- Parameters:
- Return type:
bool
- PySide2.QtCore.QSizeF.scale(s, mode)¶
- Parameters:
mode –
AspectRatioMode
This is an overloaded function.
Scales the size to a rectangle with the given
size
, according to the specifiedmode
.
- PySide2.QtCore.QSizeF.scale(w, h, mode)
- Parameters:
w – float
h – float
mode –
AspectRatioMode
Scales the size to a rectangle with the given
width
andheight
, according to the specifiedmode
.If
mode
isIgnoreAspectRatio
, the size is set to (width
,height
).If
mode
isKeepAspectRatio
, the current size is scaled to a rectangle as large as possible inside (width
,height
), preserving the aspect ratio.If
mode
isKeepAspectRatioByExpanding
, the current size is scaled to a rectangle as small as possible outside (width
,height
), preserving the aspect ratio.
Example:
t1 = QSizeF(10, 12) t1.scale(60, 60, Qt.IgnoreAspectRatio) # t1 is (60, 60) t2 = QSizeF(10, 12) t2.scale(60, 60, Qt.KeepAspectRatio) # t2 is (50, 60) t3 = QSizeF(10, 12) t3.scale(60, 60, Qt.KeepAspectRatioByExpanding) # t3 is (60, 72)
See also
- PySide2.QtCore.QSizeF.scaled(w, h, mode)¶
- Parameters:
w – float
h – float
mode –
AspectRatioMode
- Return type:
Returns a size scaled to a rectangle with the given
width
andheight
, according to the specifiedmode
.See also
- PySide2.QtCore.QSizeF.scaled(s, mode)
- Parameters:
mode –
AspectRatioMode
- Return type:
This is an overloaded function.
Returns a size scaled to a rectangle with the given size
s
, according to the specifiedmode
.
- PySide2.QtCore.QSizeF.setHeight(h)¶
- Parameters:
h – float
Sets the height to the given
height
.See also
height()
rheight()
setWidth()
- PySide2.QtCore.QSizeF.setWidth(w)¶
- Parameters:
w – float
Sets the width to the given
width
.See also
width()
rwidth()
setHeight()
- PySide2.QtCore.QSizeF.shrunkBy(m)¶
- Parameters:
- Return type:
- PySide2.QtCore.QSizeF.toSize()¶
- Return type:
Returns an integer based copy of this size.
Note that the coordinates in the returned size will be rounded to the nearest integer.
See also
QSizeF()
- PySide2.QtCore.QSizeF.toTuple()¶
- Return type:
object
- PySide2.QtCore.QSizeF.transpose()¶
Swaps the width and height values.
See also
- PySide2.QtCore.QSizeF.transposed()¶
- Return type:
Returns the size with width and height values swapped.
See also
- PySide2.QtCore.QSizeF.width()¶
- Return type:
float
Returns the width.
See also
© 2022 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.