QCursor¶
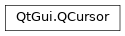
Synopsis¶
Functions¶
Static functions¶
Detailed Description¶
This class is mainly used to create mouse cursors that are associated with particular widgets and to get and set the position of the mouse cursor.
Qt has a number of standard cursor shapes, but you can also make custom cursor shapes based on a
QBitmap
, a mask and a hotspot.To associate a cursor with a widget, use
setCursor()
. To associate a cursor with all widgets (normally for a short period of time), usesetOverrideCursor()
.To set a cursor shape use
setShape()
or use theQCursor
constructor which takes the shape as argument, or you can use one of the predefined cursors defined in theCursorShape
enum.If you want to create a cursor with your own bitmap, either use the
QCursor
constructor which takes a bitmap and a mask or the constructor which takes a pixmap as arguments.To set or get the position of the mouse cursor use the static methods
pos()
andsetPos()
.Note
It is possible to create a
QCursor
beforeQGuiApplication
, but it is not useful except as a place-holder for a realQCursor
created afterQGuiApplication
. Attempting to use aQCursor
that was created beforeQGuiApplication
will result in a crash.
A Note for X11 Users¶
On X11, Qt supports the Xcursor library, which allows for full color icon themes. The table below shows the cursor name used for each
CursorShape
value. If a cursor cannot be found using the name shown below, a standard X11 cursor will be used instead. Note: X11 does not provide appropriate cursors for all possibleCursorShape
values. It is possible that some cursors will be taken from the Xcursor theme, while others will use an internal bitmap cursor.
Shape
CursorShape
ValueCursor Name
Shape
CursorShape
ValueCursor Name
ArrowCursor
left_ptr
SizeVerCursor
size_ver
UpArrowCursor
up_arrow
SizeHorCursor
size_hor
CrossCursor
cross
SizeBDiagCursor
size_bdiag
IBeamCursor
ibeam
SizeFDiagCursor
size_fdiag
WaitCursor
wait
SizeAllCursor
size_all
BusyCursor
left_ptr_watch
SplitVCursor
split_v
ForbiddenCursor
forbidden
SplitHCursor
split_h
PointingHandCursor
pointing_hand
OpenHandCursor
openhand
WhatsThisCursor
whats_this
ClosedHandCursor
closedhand
DragMoveCursor
dnd-move
ormove
DragCopyCursor
dnd-copy
orcopy
DragLinkCursor
dnd-link
orlink
See also
QWidget
GUI Design Handbook: Cursors
- class PySide2.QtGui.QCursor¶
PySide2.QtGui.QCursor(shape)
PySide2.QtGui.QCursor(bitmap, mask[, hotX=-1[, hotY=-1]])
PySide2.QtGui.QCursor(cursor)
PySide2.QtGui.QCursor(pixmap[, hotX=-1[, hotY=-1]])
- param mask:
- param shape:
- param hotX:
int
- param cursor:
- param hotY:
int
- param bitmap:
- param pixmap:
Constructs a cursor with the default arrow shape.
Constructs a cursor with the specified
shape
.See
CursorShape
for a list of shapes.See also
Constructs a custom bitmap cursor.
bitmap
andmask
make up the bitmap.hotX
andhotY
define the cursor’s hot spot.If
hotX
is negative, it is set to thebitmap().width()/2
. IfhotY
is negative, it is set to thebitmap().height()/2
.The cursor
bitmap
(B) andmask
(M) bits are combined like this:B=1 and M=1 gives black.
B=0 and M=1 gives white.
B=0 and M=0 gives transparent.
B=1 and M=0 gives an XOR’d result under Windows, undefined results on all other platforms.
Use the global Qt color
color0
to draw 0-pixels andcolor1
to draw 1-pixels in the bitmaps.Valid cursor sizes depend on the display hardware (or the underlying window system). We recommend using 32 x 32 cursors, because this size is supported on all platforms. Some platforms also support 16 x 16, 48 x 48, and 64 x 64 cursors.
See also
QBitmap()
setMask()
Constructs a custom pixmap cursor.
pixmap
is the image. It is usual to give it a mask (set usingsetMask()
).hotX
andhotY
define the cursor’s hot spot.If
hotX
is negative, it is set to thepixmap().width()/2
. IfhotY
is negative, it is set to thepixmap().height()/2
.Valid cursor sizes depend on the display hardware (or the underlying window system). We recommend using 32 x 32 cursors, because this size is supported on all platforms. Some platforms also support 16 x 16, 48 x 48, and 64 x 64 cursors.
See also
QPixmap()
setMask()
- PySide2.QtGui.QCursor.bitmap()¶
- Return type:
Note
This function is deprecated.
New code should use the other overload which returns
QBitmap
by-value.Returns the cursor bitmap, or
None
if it is one of the standard cursors.
- PySide2.QtGui.QCursor.hotSpot()¶
- Return type:
Returns the cursor hot spot, or (0, 0) if it is one of the standard cursors.
- PySide2.QtGui.QCursor.mask()¶
- Return type:
Note
This function is deprecated.
New code should use the other overload which returns
QBitmap
by-value.Returns the cursor bitmap mask, or
None
if it is one of the standard cursors.
- PySide2.QtGui.QCursor.__ne__(rhs)¶
- Parameters:
rhs –
PySide2.QtGui.QCursor
- Return type:
bool
- PySide2.QtGui.QCursor.__eq__(rhs)¶
- Parameters:
rhs –
PySide2.QtGui.QCursor
- Return type:
bool
- PySide2.QtGui.QCursor.pixmap()¶
- Return type:
Returns the cursor pixmap. This is only valid if the cursor is a pixmap cursor.
- static PySide2.QtGui.QCursor.pos()¶
- Return type:
Returns the position of the cursor (hot spot) of the primary screen in global screen coordinates.
You can call
mapFromGlobal()
to translate it to widget coordinates.Note
The position is queried from the windowing system. If mouse events are generated via other means (e.g., via QWindowSystemInterface in a unit test), those fake mouse moves will not be reflected in the returned value.
Note
On platforms where there is no windowing system or cursors are not available, the returned position is based on the mouse move events generated via QWindowSystemInterface.
See also
setPos()
mapFromGlobal()
mapToGlobal()
primaryScreen()
- static PySide2.QtGui.QCursor.pos(screen)
- Parameters:
screen –
PySide2.QtGui.QScreen
- Return type:
Returns the position of the cursor (hot spot) of the
screen
in global screen coordinates.You can call
mapFromGlobal()
to translate it to widget coordinates.See also
setPos()
mapFromGlobal()
mapToGlobal()
- static PySide2.QtGui.QCursor.setPos(screen, p)¶
- Parameters:
screen –
PySide2.QtGui.QScreen
- static PySide2.QtGui.QCursor.setPos(screen, x, y)
- Parameters:
screen –
PySide2.QtGui.QScreen
x – int
y – int
Moves the cursor (hot spot) of the
screen
to the global screen position (x
,y
).You can call
mapToGlobal()
to translate widget coordinates to global screen coordinates.Note
Calling this function results in changing the cursor position through the windowing system. The windowing system will typically respond by sending mouse events to the application’s window. This means that the usage of this function should be avoided in unit tests and everywhere where fake mouse events are being injected via QWindowSystemInterface because the windowing system’s mouse state (with regards to buttons for example) may not match the state in the application-generated events.
Note
On platforms where there is no windowing system or cursors are not available, this function may do nothing.
See also
pos()
mapFromGlobal()
mapToGlobal()
- static PySide2.QtGui.QCursor.setPos(p)
- Parameters:
This is an overloaded function.
Moves the cursor (hot spot) to the global screen position at point
p
.
- static PySide2.QtGui.QCursor.setPos(x, y)
- Parameters:
x – int
y – int
Moves the cursor (hot spot) of the primary screen to the global screen position (
x
,y
).You can call
mapToGlobal()
to translate widget coordinates to global screen coordinates.See also
pos()
mapFromGlobal()
mapToGlobal()
primaryScreen()
- PySide2.QtGui.QCursor.setShape(newShape)¶
- Parameters:
newShape –
CursorShape
Sets the cursor to the shape identified by
shape
.See
CursorShape
for the list of cursor shapes.See also
- PySide2.QtGui.QCursor.shape()¶
- Return type:
Returns the cursor shape identifier.
See also
- PySide2.QtGui.QCursor.swap(other)¶
- Parameters:
other –
PySide2.QtGui.QCursor
Swaps this cursor with the
other
cursor.
© 2022 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.