QTextBlock¶
The
QTextBlock
class provides a container for text fragments in aQTextDocument
. More…
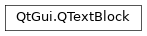
Synopsis¶
Functions¶
def
__eq__
(o)def
__iter__
()def
__lt__
(o)def
__ne__
(o)def
begin
()def
blockFormat
()def
blockFormatIndex
()def
blockNumber
()def
charFormat
()def
charFormatIndex
()def
clearLayout
()def
contains
(position)def
document
()def
end
()def
firstLineNumber
()def
fragmentIndex
()def
isValid
()def
isVisible
()def
layout
()def
length
()def
lineCount
()def
next
()def
position
()def
previous
()def
revision
()def
setLineCount
(count)def
setRevision
(rev)def
setUserData
(data)def
setUserState
(state)def
setVisible
(visible)def
text
()def
textDirection
()def
textFormats
()def
textList
()def
userData
()def
userState
()
Detailed Description¶
A text block encapsulates a block or paragraph of text in a
QTextDocument
.QTextBlock
provides read-only access to the block/paragraph structure of QTextDocuments. It is mainly of use if you want to implement your own layouts for the visual representation of aQTextDocument
, or if you want to iterate over a document and write out the contents in your own custom format.Text blocks are created by their parent documents. If you need to create a new text block, or modify the contents of a document while examining its contents, use the cursor-based interface provided by
QTextCursor
instead.Each text block is located at a specific
position()
in adocument()
. The contents of the block can be obtained by using thetext()
function. Thelength()
function determines the block’s size within the document (including formatting characters). The visual properties of the block are determined by its textlayout()
, itscharFormat()
, and itsblockFormat()
.The
next()
andprevious()
functions enable iteration over consecutive valid blocks in a document under the condition that the document is not modified by other means during the iteration process. Note that, although blocks are returned in sequence, adjacent blocks may come from different places in the document structure. The validity of a block can be determined by callingisValid()
.
QTextBlock
provides comparison operators to make it easier to work with blocks:operator==()
compares two block for equality,operator!=()
compares two blocks for inequality, andoperator
determines whether a block precedes another in the same document.![]()
See also
- class PySide2.QtGui.QTextBlock¶
PySide2.QtGui.QTextBlock(o)
- param o:
Copies the
other
text block’s attributes to this text block.
- PySide2.QtGui.QTextBlock.__iter__()¶
- Return type:
object
- PySide2.QtGui.QTextBlock.begin()¶
- Return type:
PySide2.QtGui.QTextBlock.iterator
Returns a text block iterator pointing to the beginning of the text block.
See also
- PySide2.QtGui.QTextBlock.blockFormat()¶
- Return type:
Returns the
QTextBlockFormat
that describes block-specific properties.See also
- PySide2.QtGui.QTextBlock.blockFormatIndex()¶
- Return type:
int
Returns an index into the document’s internal list of block formats for the text block’s format.
See also
- PySide2.QtGui.QTextBlock.blockNumber()¶
- Return type:
int
Returns the number of this block, or -1 if the block is invalid.
See also
- PySide2.QtGui.QTextBlock.charFormat()¶
- Return type:
Returns the
QTextCharFormat
that describes the block’s character format. The block’s character format is used when inserting text into an empty block.See also
- PySide2.QtGui.QTextBlock.charFormatIndex()¶
- Return type:
int
Returns an index into the document’s internal list of character formats for the text block’s character format.
See also
- PySide2.QtGui.QTextBlock.clearLayout()¶
Clears the
QTextLayout
that is used to lay out and display the block’s contents.See also
- PySide2.QtGui.QTextBlock.contains(position)¶
- Parameters:
position – int
- Return type:
bool
Returns
true
if the givenposition
is located within the text block; otherwise returnsfalse
.
- PySide2.QtGui.QTextBlock.document()¶
- Return type:
Returns the text document this text block belongs to, or
None
if the text block does not belong to any document.
- PySide2.QtGui.QTextBlock.end()¶
- Return type:
PySide2.QtGui.QTextBlock.iterator
Returns a text block iterator pointing to the end of the text block.
See also
- PySide2.QtGui.QTextBlock.firstLineNumber()¶
- Return type:
int
Returns the first line number of this block, or -1 if the block is invalid. Unless the layout supports it, the line number is identical to the block number.
See also
- PySide2.QtGui.QTextBlock.fragmentIndex()¶
- Return type:
int
- PySide2.QtGui.QTextBlock.isValid()¶
- Return type:
bool
Returns
true
if this text block is valid; otherwise returnsfalse
.
- PySide2.QtGui.QTextBlock.isVisible()¶
- Return type:
bool
Returns
true
if the block is visible; otherwise returnsfalse
.See also
- PySide2.QtGui.QTextBlock.layout()¶
- Return type:
Returns the
QTextLayout
that is used to lay out and display the block’s contents.Note that the returned
QTextLayout
object can only be modified from the documentChanged implementation of aQAbstractTextDocumentLayout
subclass. Any changes applied from the outside cause undefined behavior.See also
- PySide2.QtGui.QTextBlock.length()¶
- Return type:
int
Returns the length of the block in characters.
Note
The length returned includes all formatting characters, for example, newline.
See also
- PySide2.QtGui.QTextBlock.lineCount()¶
- Return type:
int
Returns the line count. Not all document layouts support this feature.
See also
- PySide2.QtGui.QTextBlock.next()¶
- Return type:
Returns the text block in the document after this block, or an empty text block if this is the last one.
Note that the next block may be in a different frame or table to this block.
See also
- PySide2.QtGui.QTextBlock.__ne__(o)¶
- Parameters:
- Return type:
bool
Returns
true
if this text block is different from theother
text block.
- PySide2.QtGui.QTextBlock.__lt__(o)¶
- Parameters:
- Return type:
bool
- PySide2.QtGui.QTextBlock.__eq__(o)¶
- Parameters:
- Return type:
bool
Returns
true
if this text block is the same as theother
text block.
- PySide2.QtGui.QTextBlock.position()¶
- Return type:
int
Returns the index of the block’s first character within the document.
- PySide2.QtGui.QTextBlock.previous()¶
- Return type:
Returns the text block in the document before this block, or an empty text block if this is the first one.
Note that the previous block may be in a different frame or table to this block.
- PySide2.QtGui.QTextBlock.revision()¶
- Return type:
int
Returns the blocks revision.
See also
- PySide2.QtGui.QTextBlock.setLineCount(count)¶
- Parameters:
count – int
Sets the line count to
count
.See also
- PySide2.QtGui.QTextBlock.setRevision(rev)¶
- Parameters:
rev – int
Sets a blocks revision to
rev
.See also
- PySide2.QtGui.QTextBlock.setUserData(data)¶
- Parameters:
Attaches the given
data
object to the text block.QTextBlockUserData
can be used to store custom settings. The ownership is passed to the underlying text document, i.e. the providedQTextBlockUserData
object will be deleted if the corresponding text block gets deleted. The user data object is not stored in the undo history, so it will not be available after undoing the deletion of a text block.For example, if you write a programming editor in an IDE, you may want to let your user set breakpoints visually in your code for an integrated debugger. In a programming editor a line of text usually corresponds to one
QTextBlock
. TheQTextBlockUserData
interface allows the developer to store data for eachQTextBlock
, like for example in which lines of the source code the user has a breakpoint set. Of course this could also be stored externally, but by storing it inside theQTextDocument
, it will for example be automatically deleted when the user deletes the associated line. It’s really just a way to store custom information in theQTextDocument
without using custom properties inQTextFormat
which would affect the undo/redo stack.See also
- PySide2.QtGui.QTextBlock.setUserState(state)¶
- Parameters:
state – int
Stores the specified
state
integer value in the text block. This may be useful for example in a syntax highlighter to store a text parsing state.See also
- PySide2.QtGui.QTextBlock.setVisible(visible)¶
- Parameters:
visible – bool
Sets the block’s visibility to
visible
.See also
- PySide2.QtGui.QTextBlock.text()¶
- Return type:
str
Returns the block’s contents as plain text.
See also
- PySide2.QtGui.QTextBlock.textDirection()¶
- Return type:
Returns the resolved text direction.
If the block has no explicit direction set, it will resolve the direction from the blocks content. Returns either
LeftToRight
orRightToLeft
.See also
layoutDirection()
isRightToLeft()
LayoutDirection
- PySide2.QtGui.QTextBlock.textFormats()¶
- Return type:
Returns the block’s text format options as a list of continuous ranges of
QTextCharFormat
. The range’s character format is used when inserting text within the range boundaries.See also
- PySide2.QtGui.QTextBlock.textList()¶
- Return type:
If the block represents a list item, returns the list that the item belongs to; otherwise returns
None
.
- PySide2.QtGui.QTextBlock.userData()¶
- Return type:
Returns a pointer to a
QTextBlockUserData
object, if one has been set withsetUserData()
, orNone
.See also
- PySide2.QtGui.QTextBlock.userState()¶
- Return type:
int
Returns the integer value previously set with
setUserState()
or -1.See also
© 2022 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.