QCborMap¶
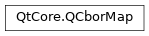
New in version 5.12.
Synopsis¶
Functions¶
def
__eq__
(other)def
__lt__
(other)def
__ne__
(other)def
clear
()def
compare
(other)def
contains
(key)def
contains
(key)def
contains
(key)def
empty
()def
isEmpty
()def
keys
()def
operator[]
(key)def
operator[]
(key)def
operator[]
(key)def
remove
(key)def
remove
(key)def
remove
(key)def
size
()def
swap
(other)def
take
(key)def
take
(key)def
take
(key)def
toCborValue
()def
toJsonObject
()def
toVariantHash
()def
toVariantMap
()def
value
(key)def
value
(key)def
value
(key)
Static functions¶
def
fromJsonObject
(o)def
fromVariantHash
(hash)def
fromVariantMap
(map)
Detailed Description¶
This class can be used to hold an associative container in CBOR, a map between a key and a value type. CBOR is the Concise Binary Object Representation, a very compact form of binary data encoding that is a superset of JSON. It was created by the IETF Constrained RESTful Environments (CoRE) WG, which has used it in many new RFCs. It is meant to be used alongside the CoAP protocol .
Unlike JSON and
QVariantMap
, CBOR map keys can be of any type, not just strings. For that reason,QCborMap
is effectively a map betweenQCborValue
keys toQCborValue
value elements.However, for all member functions that take a key parameter,
QCborMap
provides overloads that will work efficiently with integers and strings. In fact, the use of integer keys is encouraged, since they occupy fewer bytes to transmit and are simpler to encode and decode. Newer protocols designed by the IETF CoRE WG to work specifically with CBOR are known to use them.
QCborMap
is not sorted, because of that, searching for keys has linear complexity (O(n)).QCborMap
actually keeps the elements in the order that they were inserted, which means that it is possible to make sorted QCborMaps by carefully inserting elements in sorted order. CBOR does not require sorting, but recommends it.
QCborMap
can also be converted to and fromQVariantMap
andQJsonObject
. However, when performing the conversion, any non-string keys will be stringified using a one-way method that the conversion back toQCborMap
will not undo.See also
QCborArray
QCborValue
QJsonDocument
QVariantMap
- class PySide2.QtCore.QCborMap¶
PySide2.QtCore.QCborMap(other)
- param other:
Constructs an empty CBOR Map object.
See also
- PySide2.QtCore.QCborMap.compare(other)¶
- Parameters:
other –
PySide2.QtCore.QCborMap
- Return type:
int
Compares this map and
other
, comparing each element in sequence, and returns an integer that indicates whether this map should be sorted prior to (if the result is negative) or afterother
(if the result is positive). If this function returns 0, the two maps are equal and contain the same elements.Note that CBOR maps are unordered, which means that two maps containing the very same pairs but in different order will still compare differently. To avoid this, it is recommended to insert elements into the map in a predictable order, such as by ascending key value. In fact, maps with keys in sorted order are required for Canonical CBOR representation.
For more information on CBOR sorting order, see
compare()
.
- PySide2.QtCore.QCborMap.contains(key)¶
- Parameters:
- Return type:
bool
- PySide2.QtCore.QCborMap.contains(key)
- Parameters:
key – str
- Return type:
bool
- PySide2.QtCore.QCborMap.contains(key)
- Parameters:
key – int
- Return type:
bool
Returns true if this map contains a key-value pair identified by key
key
. CBOR recommends using integer keys, since they occupy less space and are simpler to encode and decode.See also
value(qint64)
operator[](qint64)
find(qint64)
remove(qint64)
contains(QLatin1String)
remove(const QString &)
remove(const QCborValue &)
- PySide2.QtCore.QCborMap.empty()¶
- Return type:
bool
Synonym for
isEmpty()
. This function is provided for compatibility with generic code that uses the Standard Library API.Returns true if this map is empty (
size()
== 0).
- static PySide2.QtCore.QCborMap.fromJsonObject(o)¶
- Parameters:
o –
QJsonObject
- Return type:
Converts all JSON items found in the
obj
object to CBOR using QCborValue::fromJson(), and returns the map composed of those elements.This conversion is lossless, as the CBOR type system is a superset of JSON’s. Moreover, the map returned by this function can be converted back to the original
obj
by usingtoJsonObject()
.
- static PySide2.QtCore.QCborMap.fromVariantHash(hash)¶
- Parameters:
hash –
- Return type:
Converts all the items in
hash
to CBOR usingfromVariant()
and returns the map composed of those elements.Conversion from
QVariant
is not completely lossless. Please see the documentation infromVariant()
for more information.
- static PySide2.QtCore.QCborMap.fromVariantMap(map)¶
- Parameters:
map –
- Return type:
Converts all the items in
map
to CBOR usingfromVariant()
and returns the map composed of those elements.Conversion from
QVariant
is not completely lossless. Please see the documentation infromVariant()
for more information.
- PySide2.QtCore.QCborMap.isEmpty()¶
- Return type:
bool
Returns true if this map is empty (that is,
size()
is 0).
- PySide2.QtCore.QCborMap.keys()¶
- Return type:
Returns a list of all keys in this map.
See also
keys()
keys()
- PySide2.QtCore.QCborMap.__ne__(other)¶
- Parameters:
other –
PySide2.QtCore.QCborMap
- Return type:
bool
Compares this map and
other
, comparing each element in sequence, and returns true if the two maps contains any different elements or elements in different orders, false otherwise.Note that CBOR maps are unordered, which means that two maps containing the very same pairs but in different order will still compare differently. To avoid this, it is recommended to insert elements into the map in a predictable order, such as by ascending key value. In fact, maps with keys in sorted order are required for Canonical CBOR representation.
For more information on CBOR equality in Qt, see,
compare()
.See also
compare()
operator==()
operator==()
operator==()
operator
- PySide2.QtCore.QCborMap.__lt__(other)¶
- Parameters:
other –
PySide2.QtCore.QCborMap
- Return type:
bool
- PySide2.QtCore.QCborMap.__eq__(other)¶
- Parameters:
other –
PySide2.QtCore.QCborMap
- Return type:
bool
Compares this map and
other
, comparing each element in sequence, and returns true if the two maps contains the same elements in the same order, false otherwise.Note that CBOR maps are unordered, which means that two maps containing the very same pairs but in different order will still compare differently. To avoid this, it is recommended to insert elements into the map in a predictable order, such as by ascending key value. In fact, maps with keys in sorted order are required for Canonical CBOR representation.
For more information on CBOR equality in Qt, see,
compare()
.See also
compare()
operator==()
operator!=()
operator
- PySide2.QtCore.QCborMap.operator[](key)
- Parameters:
- Return type:
- PySide2.QtCore.QCborMap.operator[](key)
- Parameters:
key – int
- Return type:
Returns the
QCborValue
element in this map that corresponds to keykey
, if there is one. CBOR recommends using integer keys, since they occupy less space and are simpler to encode and decode.If the map does not contain key
key
, this function returns aQCborValue
containing an undefined value. For that reason, it is not possible with this function to tell apart the situation where the key was not present from the situation where the key was mapped to an undefined value.If the map contains more than one key equal to
key
, it is undefined which one this function will return.QCborMap
does not allow inserting duplicate keys, but it is possible to create such a map by decoding a CBOR stream with them. They are usually not permitted and having duplicate keys is usually an indication of a problem in the sender.operator[](
QLatin1String
), operator[](constQString
&), operator[](const QCborOperator[] &)See also
value(qint64)
find(qint64)
constFind(qint64)
remove(qint64)
contains(qint64)
- PySide2.QtCore.QCborMap.operator[](key)
- Parameters:
key – str
- Return type:
- PySide2.QtCore.QCborMap.remove(key)¶
- Parameters:
- PySide2.QtCore.QCborMap.remove(key)
- Parameters:
key – str
- PySide2.QtCore.QCborMap.remove(key)
- Parameters:
key – int
Removes the key
key
and the corresponding value from the map, if it is found. If the map contains no such key, this function does nothing.If the map contains more than one key equal to
key
, it is undefined which one this function will remove.QCborMap
does not allow inserting duplicate keys, but it is possible to create such a map by decoding a CBOR stream with them. They are usually not permitted and having duplicate keys is usually an indication of a problem in the sender.remove(
QLatin1String
), remove(constQString
&), remove(constQCborValue
&)See also
value(qint64)
operator[](qint64)
find(qint64)
contains(qint64)
- PySide2.QtCore.QCborMap.size()¶
- Return type:
long long
Returns the number of elements in this map.
See also
- PySide2.QtCore.QCborMap.swap(other)¶
- Parameters:
other –
PySide2.QtCore.QCborMap
Swaps the contents of this map and
other
.
- PySide2.QtCore.QCborMap.take(key)¶
- Parameters:
- Return type:
- PySide2.QtCore.QCborMap.take(key)
- Parameters:
key – str
- Return type:
- PySide2.QtCore.QCborMap.take(key)
- Parameters:
key – int
- Return type:
Removes the key
key
and the corresponding value from the map and returns the value, if it is found. If the map contains no such key, this function does nothing.If the map contains more than one key equal to
key
, it is undefined which one this function will remove.QCborMap
does not allow inserting duplicate keys, but it is possible to create such a map by decoding a CBOR stream with them. They are usually not permitted and having duplicate keys is usually an indication of a problem in the sender.See also
value(qint64)
operator[](qint64)
find(qint64)
contains(qint64)
take(QLatin1String)
take(const QString &)
take(const QCborValue &)
insert()
- PySide2.QtCore.QCborMap.toCborValue()¶
- Return type:
Explicitly constructs a
QCborValue
object that represents this map. This function is usually not necessary sinceQCborValue
has a constructor forQCborMap
, so the conversion is implicit.Converting
QCborMap
toQCborValue
allows it to be used in any context where QCborValues can be used, including as keys and mapped types inQCborMap
, as well astoCbor()
.See also
QCborValue(const QCborMap &)
- PySide2.QtCore.QCborMap.toJsonObject()¶
- Return type:
QJsonObject
Recursively converts every
QCborValue
value in this map to JSON usingtoJsonValue()
and creates a string key for all keys that aren’t strings, then returns the correspondingQJsonObject
composed of those associations.Please note that CBOR contains a richer and wider type set than JSON, so some information may be lost in this conversion. For more details on what conversions are applied, see
toJsonValue()
.
Map key conversion to string¶
JSON objects are defined as having string keys, unlike CBOR, so the conversion of a
QCborMap
toQJsonObject
will imply a step of “stringification” of the key values. The conversion will use the special handling of tags and extended types from above and will also convert the rest of the types as follows:
Type
Transformation
Bool
“true” and “false”
Null
“null”
Undefined
“undefined”
Integer
The decimal string form of the number
Double
The decimal string form of the number
Byte array
Unless tagged differently (see above), encoded as Base64url
Array
Replaced by the compact form of its
Diagnostic notation
Map
Replaced by the compact form of its
Diagnostic notation
Tags and extended types
Tag number is dropped and the tagged value is converted to string
- PySide2.QtCore.QCborMap.toVariantHash()¶
- Return type:
Converts the CBOR values to
QVariant
usingtoVariant()
and “stringifies” all the CBOR keys in this map, returning theQVariantHash
that results from that association list.QVariantMaps have string keys, unlike CBOR, so the conversion of a
QCborMap
toQVariantMap
will imply a step of “stringification” of the key values. SeetoJsonObject()
for details.In addition, the conversion to
QVariant
is not completely lossless. Please see the documentation intoVariant()
for more information.
- PySide2.QtCore.QCborMap.toVariantMap()¶
- Return type:
Converts the CBOR values to
QVariant
usingtoVariant()
and “stringifies” all the CBOR keys in this map, returning theQVariantMap
that results from that association list.QVariantMaps have string keys, unlike CBOR, so the conversion of a
QCborMap
toQVariantMap
will imply a step of “stringification” of the key values. SeetoJsonObject()
for details.In addition, the conversion to
QVariant
is not completely lossless. Please see the documentation intoVariant()
for more information.
- PySide2.QtCore.QCborMap.value(key)¶
- Parameters:
- Return type:
- PySide2.QtCore.QCborMap.value(key)
- Parameters:
key – str
- Return type:
- PySide2.QtCore.QCborMap.value(key)
- Parameters:
key – int
- Return type:
Returns the
QCborValue
element in this map that corresponds to keykey
, if there is one. CBOR recommends using integer keys, since they occupy less space and are simpler to encode and decode.If the map does not contain key
key
, this function returns aQCborValue
containing an undefined value. For that reason, it is not possible with this function to tell apart the situation where the key was not present from the situation where the key was mapped to an undefined value.If the map contains more than one key equal to
key
, it is undefined which one the return from function will reference.QCborMap
does not allow inserting duplicate keys, but it is possible to create such a map by decoding a CBOR stream with them. They are usually not permitted and having duplicate keys is usually an indication of a problem in the sender.value(
QLatin1String
), value(constQString
&), value(constQCborValue
&)See also
operator[](qint64)
find(qint64)
constFind(qint64)
remove(qint64)
contains(qint64)
© 2022 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.