QCborArray¶
The
QCborArray
class is used to hold an array of CBOR elements. More…
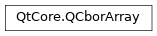
New in version 5.12.
Synopsis¶
Functions¶
def
__add__
(v)def
__eq__
(other)def
__iadd__
(v)def
__lshift__
(v)def
__lt__
(other)def
__ne__
(other)def
append
(value)def
append
(value)def
at
(i)def
clear
()def
compare
(other)def
contains
(value)def
empty
()def
first
()def
insert
(i, value)def
insert
(i, value)def
isEmpty
()def
last
()def
operator[]
(i)def
pop_back
()def
pop_front
()def
prepend
(value)def
prepend
(value)def
push_back
(t)def
push_front
(t)def
removeAt
(i)def
removeFirst
()def
removeLast
()def
size
()def
swap
(other)def
takeAt
(i)def
takeFirst
()def
takeLast
()def
toCborValue
()def
toJsonArray
()def
toVariantList
()
Static functions¶
def
fromJsonArray
(array)def
fromStringList
(list)def
fromVariantList
(list)
Detailed Description¶
This class can be used to hold one sequential container in CBOR (an array). CBOR is the Concise Binary Object Representation, a very compact form of binary data encoding that is a superset of JSON. It was created by the IETF Constrained RESTful Environments (CoRE) WG, which has used it in many new RFCs. It is meant to be used alongside the CoAP protocol .
QCborArray
is very similar toQVariantList
andQJsonArray
and its API is almost identical to those two classes. It can also be converted to and from those two, though there may be loss of information in some conversions.See also
QCborValue
QCborMap
QJsonArray
QList
QVector
- class PySide2.QtCore.QCborArray¶
PySide2.QtCore.QCborArray(other)
- param other:
Constructs an empty
QCborArray
.
- PySide2.QtCore.QCborArray.append(value)¶
- Parameters:
value –
PySide2.QtCore.QCborValue
- PySide2.QtCore.QCborArray.append(value)
- Parameters:
value –
PySide2.QtCore.QCborValue
- PySide2.QtCore.QCborArray.at(i)¶
- Parameters:
i –
long long
- Return type:
Returns the
QCborValue
element at positioni
in the array.If the array is smaller than
i
elements, this function returns aQCborValue
containing an undefined value. For that reason, it is not possible with this function to tell apart the situation where the array is not large enough from the case where the array starts with an undefined value.
- PySide2.QtCore.QCborArray.compare(other)¶
- Parameters:
other –
PySide2.QtCore.QCborArray
- Return type:
int
Compares this array and
other
, comparing each element in sequence, and returns an integer that indicates whether this array should be sorted before (if the result is negative) or afterother
(if the result is positive). If this function returns 0, the two arrays are equal and contain the same elements.For more information on CBOR sorting order, see
compare()
.
- PySide2.QtCore.QCborArray.contains(value)¶
- Parameters:
value –
PySide2.QtCore.QCborValue
- Return type:
bool
Returns true if this array contains an element that is equal to
value
.
- PySide2.QtCore.QCborArray.empty()¶
- Return type:
bool
Synonym for
isEmpty()
. This function is provided for compatibility with generic code that uses the Standard Library API.Returns true if this array is empty (
size()
== 0).
- PySide2.QtCore.QCborArray.first()¶
- Return type:
Returns the first
QCborValue
of this array.If the array is empty, this function returns a
QCborValue
containing an undefined value. For that reason, it is not possible with this function to tell apart the situation where the array is not large enough from the case where the array ends with an undefined value.
- static PySide2.QtCore.QCborArray.fromJsonArray(array)¶
- Parameters:
array –
PySide2.QtCore.QJsonArray
- Return type:
Converts all JSON items found in the
array
array to CBOR using QCborValue::fromJson(), and returns the CBOR array composed of those elements.This conversion is lossless, as the CBOR type system is a superset of JSON’s. Moreover, the array returned by this function can be converted back to the original
array
by usingtoJsonArray()
.
- static PySide2.QtCore.QCborArray.fromStringList(list)¶
- Parameters:
list – list of strings
- Return type:
Returns a
QCborArray
containing all the strings found in thelist
list.See also
- static PySide2.QtCore.QCborArray.fromVariantList(list)¶
- Parameters:
list –
- Return type:
Converts all the items in the
list
to CBOR usingfromVariant()
and returns the array composed of those elements.Conversion from
QVariant
is not completely lossless. Please see the documentation infromVariant()
for more information.
- PySide2.QtCore.QCborArray.insert(i, value)¶
- Parameters:
i –
long long
value –
PySide2.QtCore.QCborValue
- PySide2.QtCore.QCborArray.insert(i, value)
- Parameters:
i –
long long
value –
PySide2.QtCore.QCborValue
- PySide2.QtCore.QCborArray.isEmpty()¶
- Return type:
bool
Returns true if this
QCborArray
is empty (that is ifsize()
is 0).
- PySide2.QtCore.QCborArray.last()¶
- Return type:
Returns the last
QCborValue
of this array.If the array is empty, this function returns a
QCborValue
containing an undefined value. For that reason, it is not possible with this function to tell apart the situation where the array is not large enough from the case where the array ends with an undefined value.
- PySide2.QtCore.QCborArray.__ne__(other)¶
- Parameters:
other –
PySide2.QtCore.QCborArray
- Return type:
bool
Compares this array and
other
, comparing each element in sequence, and returns true if the two arrays’ contents are different, false otherwise.For more information on CBOR equality in Qt, see,
compare()
.See also
compare()
operator==()
operator==()
operator==()
operator
- PySide2.QtCore.QCborArray.__add__(v)¶
- Parameters:
- Return type:
Returns a new
QCborArray
containing the same elements as this array, plusv
appended as the last element.See also
operator+=()
operator
append()
- PySide2.QtCore.QCborArray.__iadd__(v)¶
- Parameters:
- Return type:
Appends
v
to this array and returns a reference to this array.
- PySide2.QtCore.QCborArray.__lt__(other)¶
- Parameters:
other –
PySide2.QtCore.QCborArray
- Return type:
bool
- PySide2.QtCore.QCborArray.__lshift__(v)¶
- Parameters:
- Return type:
- PySide2.QtCore.QCborArray.__eq__(other)¶
- Parameters:
other –
PySide2.QtCore.QCborArray
- Return type:
bool
Compares this array and
other
, comparing each element in sequence, and returns true if both arrays contains the same elements, false otherwise.For more information on CBOR equality in Qt, see,
compare()
.See also
compare()
operator==()
operator==()
operator!=()
operator
- PySide2.QtCore.QCborArray.operator[](i)
- Parameters:
i –
long long
- Return type:
Returns the
QCborValue
element at positioni
in the array.If the array is smaller than
i
elements, this function returns aQCborValue
containing an undefined value. For that reason, it is not possible with this function to tell apart the situation where the array is not large enough from the case where the array contains an undefined value at positioni
.
- PySide2.QtCore.QCborArray.pop_back()¶
Synonym for
removeLast()
. This function is provided for compatibility with generic code that uses the Standard Library API.Removes the last element of this array. The array must not be empty before the removal
- PySide2.QtCore.QCborArray.pop_front()¶
Synonym for
removeFirst()
. This function is provided for compatibility with generic code that uses the Standard Library API.Removes the first element of this array. The array must not be empty before the removal
- PySide2.QtCore.QCborArray.prepend(value)¶
- Parameters:
value –
PySide2.QtCore.QCborValue
- PySide2.QtCore.QCborArray.prepend(value)
- Parameters:
value –
PySide2.QtCore.QCborValue
- PySide2.QtCore.QCborArray.push_back(t)¶
- Parameters:
Synonym for
append()
. This function is provided for compatibility with generic code that uses the Standard Library API.Appends the element
t
to this array.See also
- PySide2.QtCore.QCborArray.push_front(t)¶
- Parameters:
Synonym for
prepend()
. This function is provided for compatibility with generic code that uses the Standard Library API.Prepends the element
t
to this array.See also
- PySide2.QtCore.QCborArray.removeAt(i)¶
- Parameters:
i –
long long
Removes the item at position
i
from the array. The array must have more thani
elements before the removal.See also
takeAt()
removeFirst()
removeLast()
at()
operator[]()
insert()
prepend()
append()
- PySide2.QtCore.QCborArray.removeFirst()¶
Removes the first item in the array, making the second element become the first. The array must not be empty before this call.
See also
removeAt()
takeFirst()
removeLast()
at()
operator[]()
insert()
prepend()
append()
- PySide2.QtCore.QCborArray.removeLast()¶
Removes the last item in the array. The array must not be empty before this call.
See also
removeAt()
takeLast()
removeFirst()
at()
operator[]()
insert()
prepend()
append()
- PySide2.QtCore.QCborArray.size()¶
- Return type:
long long
Returns the size of this array.
See also
- PySide2.QtCore.QCborArray.swap(other)¶
- Parameters:
other –
PySide2.QtCore.QCborArray
Swaps the contents of this object and
other
.
- PySide2.QtCore.QCborArray.takeAt(i)¶
- Parameters:
i –
long long
- Return type:
Removes the item at position
i
from the array and returns it. The array must have more thani
elements before the removal.See also
removeAt()
removeFirst()
removeLast()
at()
operator[]()
insert()
prepend()
append()
- PySide2.QtCore.QCborArray.takeFirst()¶
- Return type:
Removes the first item in the array and returns it, making the second element become the first. The array must not be empty before this call.
See also
takeAt()
removeFirst()
removeLast()
at()
operator[]()
insert()
prepend()
append()
- PySide2.QtCore.QCborArray.takeLast()¶
- Return type:
Removes the last item in the array and returns it. The array must not be empty before this call.
See also
takeAt()
removeLast()
removeFirst()
at()
operator[]()
insert()
prepend()
append()
- PySide2.QtCore.QCborArray.toCborValue()¶
- Return type:
Explicitly construcuts a
QCborValue
object that represents this array. This function is usually not necessary sinceQCborValue
has a constructor forQCborArray
, so the conversion is implicit.Converting
QCborArray
toQCborValue
allows it to be used in any context where QCborValues can be used, including as items in QCborArrays and as keys and mapped types inQCborMap
. Converting an array toQCborValue
allows access totoCbor()
.See also
QCborValue(const QCborArray &)
- PySide2.QtCore.QCborArray.toJsonArray()¶
- Return type:
Recursively converts every
QCborValue
element in this array to JSON usingtoJsonValue()
and returns the correspondingQJsonArray
composed of those elements.Please note that CBOR contains a richer and wider type set than JSON, so some information may be lost in this conversion. For more details on what conversions are applied, see
toJsonValue()
.
- PySide2.QtCore.QCborArray.toVariantList()¶
- Return type:
Recursively converts each
QCborValue
in this array usingtoVariant()
and returns theQVariantList
composed of the converted items.Conversion to
QVariant
is not completely lossless. Please see the documentation intoVariant()
for more information.
© 2022 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.