QMutexLocker¶
The
QMutexLocker
class is a convenience class that simplifies locking and unlocking mutexes. More…
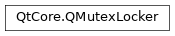
Synopsis¶
Functions¶
Detailed Description¶
Locking and unlocking a
QMutex
in complex functions and statements or in exception handling code is error-prone and difficult to debug.QMutexLocker
can be used in such situations to ensure that the state of the mutex is always well-defined.
QMutexLocker
should be created within a function where aQMutex
needs to be locked. The mutex is locked whenQMutexLocker
is created. You can unlock and relock the mutex withunlock()
andrelock()
. If locked, the mutex will be unlocked when theQMutexLocker
is destroyed.For example, this complex function locks a
QMutex
upon entering the function and unlocks the mutex at all the exit points:def complexFunction(flag): mutex.lock() retVal = 0 if flag == 0 or flag == 1: mutex.unlock() return moreComplexFunction(flag) elif flag == 2: status = anotherFunction() if status < 0: mutex.unlock() return -2 retVal = status + flag else: if flag > 10: mutex.unlock() return -1 mutex.unlock() return retValThis example function will get more complicated as it is developed, which increases the likelihood that errors will occur.
Using
QMutexLocker
greatly simplifies the code, and makes it more readable:def complexFunction(flag): locker = QMutexLocker(mutex) retVal = 0 if flag == 0 or flag == 1: return moreComplexFunction(flag) elif flag == 2: status = anotherFunction() if status < 0: return -2 retVal = status + flag else: if flag > 10: return -1 return retValNow, the mutex will always be unlocked when the
QMutexLocker
object is destroyed (when the function returns sincelocker
is an auto variable).The same principle applies to code that throws and catches exceptions. An exception that is not caught in the function that has locked the mutex has no way of unlocking the mutex before the exception is passed up the stack to the calling function.
QMutexLocker
also provides amutex()
member function that returns the mutex on which theQMutexLocker
is operating. This is useful for code that needs access to the mutex, such aswait()
. For example:class SignalWaiter: def __init__(mutex): self.locker = mutex def waitForSignal(): # ... while not signalled: waitCondition.wait(self.locker.mutex()) # ...See also
- class PySide2.QtCore.QMutexLocker(m)¶
PySide2.QtCore.QMutexLocker(m)
- param m:
Constructs a
QMutexLocker
and locksmutex
. The mutex will be unlocked (unlock()
called) when theQMutexLocker
is destroyed. Ifmutex
isNone
,QMutexLocker
does nothing.See also
lock()
- PySide2.QtCore.QMutexLocker.__enter__()¶
- PySide2.QtCore.QMutexLocker.__exit__(arg__1, arg__2, arg__3)¶
- Parameters:
arg__1 – object
arg__2 – object
arg__3 – object
- PySide2.QtCore.QMutexLocker.mutex()¶
- Return type:
Returns the mutex on which the
QMutexLocker
is operating.
© 2022 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.