QVector4D¶
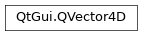
New in version 4.6.
Synopsis¶
Functions¶
def
__add__
(, v2)def
__div__
(, divisor)def
__div__
(, divisor)def
__eq__
(, v2)def
__iadd__
(vector)def
__idiv__
(divisor)def
__idiv__
(vector)def
__imul__
(factor)def
__imul__
(vector)def
__isub__
(vector)def
__mul__
(, factor)def
__mul__
(, matrix)def
__mul__
(, v2)def
__mul__
(factor)def
__mul__
(matrix)def
__ne__
(, v2)def
__reduce__
()def
__repr__
()def
__sub__
()def
__sub__
(, v2)def
isNull
()def
length
()def
lengthSquared
()def
normalize
()def
normalized
()def
operator[]
(i)def
setW
(w)def
setX
(x)def
setY
(y)def
setZ
(z)def
toPoint
()def
toPointF
()def
toTuple
()def
toVector2D
()def
toVector2DAffine
()def
toVector3D
()def
toVector3DAffine
()def
w
()def
x
()def
y
()def
z
()
Static functions¶
def
dotProduct
(v1, v2)
Detailed Description¶
The
QVector4D
class can also be used to represent vertices in 4D space. We therefore do not need to provide a separate vertex class.See also
- class PySide2.QtGui.QVector4D¶
PySide2.QtGui.QVector4D(point)
PySide2.QtGui.QVector4D(point)
PySide2.QtGui.QVector4D(vector)
PySide2.QtGui.QVector4D(vector, zpos, wpos)
PySide2.QtGui.QVector4D(vector)
PySide2.QtGui.QVector4D(vector, wpos)
PySide2.QtGui.QVector4D(xpos, ypos, zpos, wpos)
- param ypos:
float
- param point:
- param wpos:
float
- param zpos:
float
- param vector:
- param xpos:
float
Constructs a null vector, i.e. with coordinates (0, 0, 0, 0).
Constructs a 4D vector from the specified 2D
vector
. The z and w coordinates are set tozpos
andwpos
respectively.See also
Constructs a 4D vector from the specified 3D
vector
. The w coordinate is set towpos
.See also
Constructs a vector with coordinates (
xpos
,ypos
,zpos
,wpos
).
- PySide2.QtGui.QVector4D.__reduce__()¶
- Return type:
object
- PySide2.QtGui.QVector4D.__repr__()¶
- Return type:
object
- static PySide2.QtGui.QVector4D.dotProduct(v1, v2)¶
- Parameters:
- Return type:
float
Returns the dot product of
v1
andv2
.
- PySide2.QtGui.QVector4D.isNull()¶
- Return type:
bool
Returns
true
if the x, y, z, and w coordinates are set to 0.0, otherwise returnsfalse
.
- PySide2.QtGui.QVector4D.length()¶
- Return type:
float
Returns the length of the vector from the origin.
See also
- PySide2.QtGui.QVector4D.lengthSquared()¶
- Return type:
float
Returns the squared length of the vector from the origin. This is equivalent to the dot product of the vector with itself.
See also
- PySide2.QtGui.QVector4D.normalize()¶
Normalizes the currect vector in place. Nothing happens if this vector is a null vector or the length of the vector is very close to 1.
See also
- PySide2.QtGui.QVector4D.normalized()¶
- Return type:
Returns the normalized unit vector form of this vector.
If this vector is null, then a null vector is returned. If the length of the vector is very close to 1, then the vector will be returned as-is. Otherwise the normalized form of the vector of length 1 will be returned.
See also
- PySide2.QtGui.QVector4D.__ne__(v2)¶
- Parameters:
- Return type:
bool
- PySide2.QtGui.QVector4D.__mul__(matrix)¶
- Parameters:
matrix –
PySide2.QtGui.QMatrix4x4
- Return type:
- PySide2.QtGui.QVector4D.__mul__(matrix)
- Parameters:
matrix –
PySide2.QtGui.QMatrix4x4
- Return type:
- PySide2.QtGui.QVector4D.__mul__(v2)
- Parameters:
- Return type:
- PySide2.QtGui.QVector4D.__mul__(factor)
- Parameters:
factor – float
- Return type:
- PySide2.QtGui.QVector4D.__mul__(factor)
- Parameters:
factor – float
- Return type:
- PySide2.QtGui.QVector4D.__imul__(vector)¶
- Parameters:
vector –
PySide2.QtGui.QVector4D
- Return type:
- PySide2.QtGui.QVector4D.__imul__(factor)
- Parameters:
factor – float
- Return type:
Multiplies this vector’s coordinates by the given
factor
, and returns a reference to this vector.See also
operator/=()
- PySide2.QtGui.QVector4D.__add__(v2)¶
- Parameters:
- Return type:
- PySide2.QtGui.QVector4D.__iadd__(vector)¶
- Parameters:
vector –
PySide2.QtGui.QVector4D
- Return type:
Adds the given
vector
to this vector and returns a reference to this vector.See also
operator-=()
- PySide2.QtGui.QVector4D.__sub__(v2)¶
- Parameters:
- Return type:
- PySide2.QtGui.QVector4D.__sub__()
- Return type:
- PySide2.QtGui.QVector4D.__isub__(vector)¶
- Parameters:
vector –
PySide2.QtGui.QVector4D
- Return type:
Subtracts the given
vector
from this vector and returns a reference to this vector.See also
operator+=()
- PySide2.QtGui.QVector4D.__div__(divisor)¶
- Parameters:
divisor –
PySide2.QtGui.QVector4D
- Return type:
- PySide2.QtGui.QVector4D.__div__(divisor)
- Parameters:
divisor – float
- Return type:
- PySide2.QtGui.QVector4D.__idiv__(vector)¶
- Parameters:
vector –
PySide2.QtGui.QVector4D
- Return type:
- PySide2.QtGui.QVector4D.__idiv__(divisor)
- Parameters:
divisor – float
- Return type:
Divides this vector’s coordinates by the given
divisor
, and returns a reference to this vector.See also
operator*=()
- PySide2.QtGui.QVector4D.__eq__(v2)¶
- Parameters:
- Return type:
bool
- PySide2.QtGui.QVector4D.operator[](i)
- Parameters:
i – int
- Return type:
float
Returns the component of the vector at index position
i
.i
must be a valid index position in the vector (i.e., 0 <=i
< 4).
- PySide2.QtGui.QVector4D.setW(w)¶
- Parameters:
w – float
Sets the w coordinate of this point to the given
w
coordinate.
- PySide2.QtGui.QVector4D.setX(x)¶
- Parameters:
x – float
Sets the x coordinate of this point to the given
x
coordinate.
- PySide2.QtGui.QVector4D.setY(y)¶
- Parameters:
y – float
Sets the y coordinate of this point to the given
y
coordinate.
- PySide2.QtGui.QVector4D.setZ(z)¶
- Parameters:
z – float
Sets the z coordinate of this point to the given
z
coordinate.
- PySide2.QtGui.QVector4D.toPoint()¶
- Return type:
Returns the
QPoint
form of this 4D vector. The z and w coordinates are dropped.See also
- PySide2.QtGui.QVector4D.toPointF()¶
- Return type:
Returns the
QPointF
form of this 4D vector. The z and w coordinates are dropped.See also
- PySide2.QtGui.QVector4D.toTuple()¶
- Return type:
object
- PySide2.QtGui.QVector4D.toVector2D()¶
- Return type:
Returns the 2D vector form of this 4D vector, dropping the z and w coordinates.
See also
- PySide2.QtGui.QVector4D.toVector2DAffine()¶
- Return type:
Returns the 2D vector form of this 4D vector, dividing the x and y coordinates by the w coordinate and dropping the z coordinate. Returns a null vector if w is zero.
See also
- PySide2.QtGui.QVector4D.toVector3D()¶
- Return type:
Returns the 3D vector form of this 4D vector, dropping the w coordinate.
See also
- PySide2.QtGui.QVector4D.toVector3DAffine()¶
- Return type:
Returns the 3D vector form of this 4D vector, dividing the x, y, and z coordinates by the w coordinate. Returns a null vector if w is zero.
See also
- PySide2.QtGui.QVector4D.w()¶
- Return type:
float
Returns the w coordinate of this point.
- PySide2.QtGui.QVector4D.x()¶
- Return type:
float
Returns the x coordinate of this point.
- PySide2.QtGui.QVector4D.y()¶
- Return type:
float
Returns the y coordinate of this point.
- PySide2.QtGui.QVector4D.z()¶
- Return type:
float
Returns the z coordinate of this point.
© 2022 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.