QMatrix4x4¶
The
QMatrix4x4
class represents a 4x4 transformation matrix in 3D space. More…
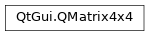
New in version 4.6.
Synopsis¶
Functions¶
def
__add__
(, m2)def
__div__
(, divisor)def
__dummy
(arg__1)def
__eq__
(other)def
__iadd__
(other)def
__idiv__
(divisor)def
__imul__
(factor)def
__imul__
(other)def
__isub__
(other)def
__mgetitem__
()def
__mul__
(, factor)def
__mul__
(, m2)def
__mul__
(factor)def
__ne__
(other)def
__reduce__
()def
__repr__
()def
__sub__
()def
__sub__
(, m2)def
column
(index)def
copyDataTo
()def
determinant
()def
fill
(value)def
flipCoordinates
()def
frustum
(left, right, bottom, top, nearPlane, farPlane)def
inverted
()def
isAffine
()def
isIdentity
()def
lookAt
(eye, center, up)def
map
(point)def
map
(point)def
map
(point)def
map
(point)def
mapRect
(rect)def
mapRect
(rect)def
mapVector
(vector)def
normalMatrix
()def
optimize
()def
ortho
(left, right, bottom, top, nearPlane, farPlane)def
ortho
(rect)def
ortho
(rect)def
perspective
(verticalAngle, aspectRatio, nearPlane, farPlane)def
rotate
(angle, vector)def
rotate
(angle, x, y[, z=0.0f])def
rotate
(quaternion)def
row
(index)def
scale
(factor)def
scale
(vector)def
scale
(x, y)def
scale
(x, y, z)def
setColumn
(index, value)def
setRow
(index, value)def
setToIdentity
()def
toAffine
()def
toTransform
()def
toTransform
(distanceToPlane)def
translate
(vector)def
translate
(x, y)def
translate
(x, y, z)def
transposed
()def
viewport
(left, bottom, width, height[, nearPlane=0.0f[, farPlane=1.0f]])def
viewport
(rect)
Detailed Description¶
The
QMatrix4x4
class in general is treated as a row-major matrix, in that the constructors andoperator()
functions take data in row-major format, as is familiar in C-style usage.Internally the data is stored as column-major format, so as to be optimal for passing to OpenGL functions, which expect column-major data.
When using these functions be aware that they return data in column-major format:
data()
constData()
See also
QVector3D
QGenericMatrix
- class PySide2.QtGui.QMatrix4x4¶
PySide2.QtGui.QMatrix4x4(matrix)
PySide2.QtGui.QMatrix4x4(transform)
PySide2.QtGui.QMatrix4x4(values)
PySide2.QtGui.QMatrix4x4(m11, m12, m13, m14, m21, m22, m23, m24, m31, m32, m33, m34, m41, m42, m43, m44)
- param m43:
float
- param m44:
float
- param m31:
float
- param m32:
float
- param m33:
float
- param m34:
float
- param transform:
- param m21:
float
- param m22:
float
- param m23:
float
- param m24:
float
- param m11:
float
- param m12:
float
- param m13:
float
- param m14:
float
- param values:
float
- param matrix:
- param m41:
float
- param m42:
float
Constructs an identity matrix.
Constructs a matrix from the given 16 floating-point
values
. The contents of the arrayvalues
is assumed to be in row-major order.If the matrix has a special type (identity, translate, scale, etc), the programmer should follow this constructor with a call to
optimize()
if they wishQMatrix4x4
to optimize further calls totranslate()
,scale()
, etc.See also
Constructs a matrix from the 16 elements
m11
,m12
,m13
,m14
,m21
,m22
,m23
,m24
,m31
,m32
,m33
,m34
,m41
,m42
,m43
, andm44
. The elements are specified in row-major order.If the matrix has a special type (identity, translate, scale, etc), the programmer should follow this constructor with a call to
optimize()
if they wishQMatrix4x4
to optimize further calls totranslate()
,scale()
, etc.See also
- PySide2.QtGui.QMatrix4x4.enum_383¶
- PySide2.QtGui.QMatrix4x4.__dummy(arg__1)¶
- Parameters:
arg__1 –
- PySide2.QtGui.QMatrix4x4.__mgetitem__()¶
- Return type:
object
- PySide2.QtGui.QMatrix4x4.__reduce__()¶
- Return type:
object
- PySide2.QtGui.QMatrix4x4.__repr__()¶
- Return type:
object
- PySide2.QtGui.QMatrix4x4.column(index)¶
- Parameters:
index – int
- Return type:
Returns the elements of column
index
as a 4D vector.See also
- PySide2.QtGui.QMatrix4x4.copyDataTo()¶
Retrieves the 16 items in this matrix and copies them to
values
in row-major order.
- PySide2.QtGui.QMatrix4x4.determinant()¶
- Return type:
double
Returns the determinant of this matrix.
- PySide2.QtGui.QMatrix4x4.fill(value)¶
- Parameters:
value – float
Fills all elements of this matrx with
value
.
- PySide2.QtGui.QMatrix4x4.flipCoordinates()¶
Flips between right-handed and left-handed coordinate systems by multiplying the y and z co-ordinates by -1. This is normally used to create a left-handed orthographic view without scaling the viewport as
ortho()
does.See also
- PySide2.QtGui.QMatrix4x4.frustum(left, right, bottom, top, nearPlane, farPlane)¶
- Parameters:
left – float
right – float
bottom – float
top – float
nearPlane – float
farPlane – float
Multiplies this matrix by another that applies a perspective frustum projection for a window with lower-left corner (
left
,bottom
), upper-right corner (right
,top
), and the specifiednearPlane
andfarPlane
clipping planes.See also
- PySide2.QtGui.QMatrix4x4.inverted()¶
- Return type:
PyTuple
Returns the inverse of this matrix. Returns the identity if this matrix cannot be inverted; i.e.
determinant()
is zero. Ifinvertible
is not null, then true will be written to that location if the matrix can be inverted; false otherwise.If the matrix is recognized as the identity or an orthonormal matrix, then this function will quickly invert the matrix using optimized routines.
See also
- PySide2.QtGui.QMatrix4x4.isAffine()¶
- Return type:
bool
Returns
true
if this matrix is affine matrix; false otherwise.An affine matrix is a 4x4 matrix with row 3 equal to (0, 0, 0, 1), e.g. no projective coefficients.
See also
- PySide2.QtGui.QMatrix4x4.isIdentity()¶
- Return type:
bool
Returns
true
if this matrix is the identity; false otherwise.See also
- PySide2.QtGui.QMatrix4x4.lookAt(eye, center, up)¶
- Parameters:
eye –
PySide2.QtGui.QVector3D
center –
PySide2.QtGui.QVector3D
Multiplies this matrix by a viewing matrix derived from an eye point. The
center
value indicates the center of the view that theeye
is looking at. Theup
value indicates which direction should be considered up with respect to theeye
.Note
The
up
vector must not be parallel to the line of sight fromeye
tocenter
.
- PySide2.QtGui.QMatrix4x4.map(point)¶
- Parameters:
point –
PySide2.QtCore.QPoint
- Return type:
- PySide2.QtGui.QMatrix4x4.map(point)
- Parameters:
point –
PySide2.QtCore.QPointF
- Return type:
- PySide2.QtGui.QMatrix4x4.map(point)
- Parameters:
point –
PySide2.QtGui.QVector3D
- Return type:
- PySide2.QtGui.QMatrix4x4.map(point)
- Parameters:
point –
PySide2.QtGui.QVector4D
- Return type:
- PySide2.QtGui.QMatrix4x4.mapRect(rect)¶
- Parameters:
rect –
PySide2.QtCore.QRect
- Return type:
- PySide2.QtGui.QMatrix4x4.mapRect(rect)
- Parameters:
rect –
PySide2.QtCore.QRectF
- Return type:
- PySide2.QtGui.QMatrix4x4.mapVector(vector)¶
- Parameters:
vector –
PySide2.QtGui.QVector3D
- Return type:
Maps
vector
by multiplying the top 3x3 portion of this matrix byvector
. The translation and projection components of this matrix are ignored.See also
- PySide2.QtGui.QMatrix4x4.normalMatrix()¶
- Return type:
Returns the normal matrix corresponding to this 4x4 transformation. The normal matrix is the transpose of the inverse of the top-left 3x3 part of this 4x4 matrix. If the 3x3 sub-matrix is not invertible, this function returns the identity.
See also
- PySide2.QtGui.QMatrix4x4.__ne__(other)¶
- Parameters:
other –
PySide2.QtGui.QMatrix4x4
- Return type:
bool
Returns
true
if this matrix is not identical toother
; false otherwise. This operator uses an exact floating-point comparison.
- PySide2.QtGui.QMatrix4x4.__mul__(factor)¶
- Parameters:
factor – float
- Return type:
- PySide2.QtGui.QMatrix4x4.__mul__(m2)
- Parameters:
- Return type:
- PySide2.QtGui.QMatrix4x4.__mul__(factor)
- Parameters:
factor – float
- Return type:
- PySide2.QtGui.QMatrix4x4.__imul__(other)¶
- Parameters:
other –
PySide2.QtGui.QMatrix4x4
- Return type:
- PySide2.QtGui.QMatrix4x4.__imul__(factor)
- Parameters:
factor – float
- Return type:
This is an overloaded function.
Multiplies all elements of this matrix by
factor
.
- PySide2.QtGui.QMatrix4x4.__add__(m2)¶
- Parameters:
- Return type:
- PySide2.QtGui.QMatrix4x4.__iadd__(other)¶
- Parameters:
other –
PySide2.QtGui.QMatrix4x4
- Return type:
Adds the contents of
other
to this matrix.
- PySide2.QtGui.QMatrix4x4.__sub__()¶
- Return type:
- PySide2.QtGui.QMatrix4x4.__sub__(m2)
- Parameters:
- Return type:
This is an overloaded function.
Returns the negation of
matrix
.
- PySide2.QtGui.QMatrix4x4.__isub__(other)¶
- Parameters:
other –
PySide2.QtGui.QMatrix4x4
- Return type:
Subtracts the contents of
other
from this matrix.
- PySide2.QtGui.QMatrix4x4.__div__(divisor)¶
- Parameters:
divisor – float
- Return type:
- PySide2.QtGui.QMatrix4x4.__idiv__(divisor)¶
- Parameters:
divisor – float
- Return type:
This is an overloaded function.
Divides all elements of this matrix by
divisor
.
- PySide2.QtGui.QMatrix4x4.__eq__(other)¶
- Parameters:
other –
PySide2.QtGui.QMatrix4x4
- Return type:
bool
Returns
true
if this matrix is identical toother
; false otherwise. This operator uses an exact floating-point comparison.
- PySide2.QtGui.QMatrix4x4.optimize()¶
Optimize the usage of this matrix from its current elements.
Some operations such as
translate()
,scale()
, androtate()
can be performed more efficiently if the matrix being modified is already known to be the identity, a previoustranslate()
, a previousscale()
, etc.Normally the
QMatrix4x4
class keeps track of this special type internally as operations are performed. However, if the matrix is modified directly withoperator()
() ordata()
, thenQMatrix4x4
will lose track of the special type and will revert to the safest but least efficient operations thereafter.By calling after directly modifying the matrix, the programmer can force
QMatrix4x4
to recover the special type if the elements appear to conform to one of the known optimized types.See also
data()
translate()
- PySide2.QtGui.QMatrix4x4.ortho(rect)¶
- Parameters:
rect –
PySide2.QtCore.QRect
- PySide2.QtGui.QMatrix4x4.ortho(rect)
- Parameters:
rect –
PySide2.QtCore.QRectF
- PySide2.QtGui.QMatrix4x4.ortho(left, right, bottom, top, nearPlane, farPlane)
- Parameters:
left – float
right – float
bottom – float
top – float
nearPlane – float
farPlane – float
Multiplies this matrix by another that applies an orthographic projection for a window with lower-left corner (
left
,bottom
), upper-right corner (right
,top
), and the specifiednearPlane
andfarPlane
clipping planes.See also
- PySide2.QtGui.QMatrix4x4.perspective(verticalAngle, aspectRatio, nearPlane, farPlane)¶
- Parameters:
verticalAngle – float
aspectRatio – float
nearPlane – float
farPlane – float
Multiplies this matrix by another that applies a perspective projection. The vertical field of view will be
verticalAngle
degrees within a window with a givenaspectRatio
that determines the horizontal field of view. The projection will have the specifiednearPlane
andfarPlane
clipping planes which are the distances from the viewer to the corresponding planes.
- PySide2.QtGui.QMatrix4x4.rotate(quaternion)¶
- Parameters:
quaternion –
PySide2.QtGui.QQuaternion
Multiples this matrix by another that rotates coordinates according to a specified
quaternion
. Thequaternion
is assumed to have been normalized.See also
- PySide2.QtGui.QMatrix4x4.rotate(angle, x, y[, z=0.0f])
- Parameters:
angle – float
x – float
y – float
z – float
This is an overloaded function.
Multiplies this matrix by another that rotates coordinates through
angle
degrees about the vector (x
,y
,z
).See also
- PySide2.QtGui.QMatrix4x4.rotate(angle, vector)
- Parameters:
angle – float
vector –
PySide2.QtGui.QVector3D
Multiples this matrix by another that rotates coordinates through
angle
degrees aboutvector
.See also
- PySide2.QtGui.QMatrix4x4.row(index)¶
- Parameters:
index – int
- Return type:
Returns the elements of row
index
as a 4D vector.
- PySide2.QtGui.QMatrix4x4.scale(vector)¶
- Parameters:
vector –
PySide2.QtGui.QVector3D
- PySide2.QtGui.QMatrix4x4.scale(factor)
- Parameters:
factor – float
This is an overloaded function.
Multiplies this matrix by another that scales coordinates by the given
factor
.See also
- PySide2.QtGui.QMatrix4x4.scale(x, y)
- Parameters:
x – float
y – float
This is an overloaded function.
Multiplies this matrix by another that scales coordinates by the components
x
, andy
.See also
- PySide2.QtGui.QMatrix4x4.scale(x, y, z)
- Parameters:
x – float
y – float
z – float
This is an overloaded function.
Multiplies this matrix by another that scales coordinates by the components
x
,y
, andz
.See also
- PySide2.QtGui.QMatrix4x4.setColumn(index, value)¶
- Parameters:
index – int
value –
PySide2.QtGui.QVector4D
Sets the elements of column
index
to the components ofvalue
.
- PySide2.QtGui.QMatrix4x4.setRow(index, value)¶
- Parameters:
index – int
value –
PySide2.QtGui.QVector4D
Sets the elements of row
index
to the components ofvalue
.See also
- PySide2.QtGui.QMatrix4x4.setToIdentity()¶
Sets this matrix to the identity.
See also
- PySide2.QtGui.QMatrix4x4.toAffine()¶
- Return type:
Note
This function is deprecated.
Use
toTransform()
instead.Returns the conventional Qt 2D affine transformation matrix that corresponds to this matrix. It is assumed that this matrix only contains 2D affine transformation elements.
See also
- PySide2.QtGui.QMatrix4x4.toTransform()¶
- Return type:
Returns the conventional Qt 2D transformation matrix that corresponds to this matrix.
The returned
QTransform
is formed by simply dropping the third row and third column of theQMatrix4x4
. This is suitable for implementing orthographic projections where the z co-ordinate should be dropped rather than projected.See also
- PySide2.QtGui.QMatrix4x4.toTransform(distanceToPlane)
- Parameters:
distanceToPlane – float
- Return type:
Returns the conventional Qt 2D transformation matrix that corresponds to this matrix.
If
distanceToPlane
is non-zero, it indicates a projection factor to use to adjust for the z co-ordinate. The value of 1024 corresponds to the projection factor used byrotate()
for the x and y axes.If
distanceToPlane
is zero, then the returnedQTransform
is formed by simply dropping the third row and third column of theQMatrix4x4
. This is suitable for implementing orthographic projections where the z co-ordinate should be dropped rather than projected.See also
- PySide2.QtGui.QMatrix4x4.translate(vector)¶
- Parameters:
vector –
PySide2.QtGui.QVector3D
Multiplies this matrix by another that translates coordinates by the components of
vector
.
- PySide2.QtGui.QMatrix4x4.translate(x, y)
- Parameters:
x – float
y – float
This is an overloaded function.
Multiplies this matrix by another that translates coordinates by the components
x
, andy
.
- PySide2.QtGui.QMatrix4x4.translate(x, y, z)
- Parameters:
x – float
y – float
z – float
This is an overloaded function.
Multiplies this matrix by another that translates coordinates by the components
x
,y
, andz
.
- PySide2.QtGui.QMatrix4x4.transposed()¶
- Return type:
Returns this matrix, transposed about its diagonal.
- PySide2.QtGui.QMatrix4x4.viewport(rect)¶
- Parameters:
rect –
PySide2.QtCore.QRectF
This is an overloaded function.
Sets up viewport transform for viewport bounded by
rect
and with near and far set to 0 and 1 respectively.
- PySide2.QtGui.QMatrix4x4.viewport(left, bottom, width, height[, nearPlane=0.0f[, farPlane=1.0f]])
- Parameters:
left – float
bottom – float
width – float
height – float
nearPlane – float
farPlane – float
Multiplies this matrix by another that performs the scale and bias transformation used by OpenGL to transform from normalized device coordinates (NDC) to viewport (window) coordinates. That is it maps points from the cube ranging over [-1, 1] in each dimension to the viewport with it’s near-lower-left corner at (
left
,bottom
,nearPlane
) and with size (width
,height
,farPlane
-nearPlane
).This matches the transform used by the fixed function OpenGL viewport transform controlled by the functions glViewport() and glDepthRange().
© 2022 The Qt Company Ltd. Documentation contributions included herein are the copyrights of their respective owners. The documentation provided herein is licensed under the terms of the GNU Free Documentation License version 1.3 as published by the Free Software Foundation. Qt and respective logos are trademarks of The Qt Company Ltd. in Finland and/or other countries worldwide. All other trademarks are property of their respective owners.