Expenses Tool Tutorial#
- In this tutorial you will learn the following concepts:
creating user interfaces programatically,
layouts and widgets,
overloading Qt classes,
connecting signal and slots,
interacting with QWidgets,
and building your own application.
- The requirements:
A simple window for the application (QMainWindow).
A table to keep track of the expenses (QTableWidget).
Two input fields to add expense information (QLineEdit).
Buttons to add information to the table, plot data, clear table, and exit the application (QPushButton).
A verification step to avoid invalid data entry.
A chart to visualize the expense data (QChart) that will be embedded in a chart view (QChartView).
Empty window#
The base structure for a QApplication is located inside the if __name__ == “__main__”: code block.
1 if __name__ == "__main__":
2 app = QApplication([])
3 # ...
4 sys.exit(app.exec())
Now, to start the development, create an empty window called MainWindow. You could do that by defining a class that inherits from QMainWindow.
1class MainWindow(QMainWindow):
2 def __init__(self):
3 super().__init__()
4 self.setWindowTitle("Tutorial")
5
6if __name__ == "__main__":
7 # Qt Application
8 app = QApplication(sys.argv)
9
10 window = MainWindow()
11 window.resize(800, 600)
12 window.show()
13
14 # Execute application
15 sys.exit(app.exec())
Now that our class is defined, create an instance of it and call show().
1class MainWindow(QMainWindow):
2 def __init__(self):
3 super().__init__()
4 self.setWindowTitle("Tutorial")
5
6if __name__ == "__main__":
7 # Qt Application
8 app = QApplication(sys.argv)
9
10 window = MainWindow()
11 window.resize(800, 600)
12 window.show()
13
14 # Execute application
15 sys.exit(app.exec())
Empty widget and data#
The QMainWindow enables us to set a central widget that will be displayed when showing the window (read more). This central widget could be another class derived from QWidget.
Additionally, you will define example data to visualize later.
1class Widget(QWidget):
2 def __init__(self):
3 super().__init__()
4
5 # Example data
6 self._data = {"Water": 24.5, "Electricity": 55.1, "Rent": 850.0,
7 "Supermarket": 230.4, "Internet": 29.99, "Bars": 21.85,
8 "Public transportation": 60.0, "Coffee": 22.45, "Restaurants": 120}
With the Widget class in place, modify MainWindow’s initialization code
1 # QWidget
2 widget = Widget()
3 # QMainWindow using QWidget as central widget
4 window = MainWindow(widget)
Window layout#
Now that the main empty window is in place, you need to start adding widgets to achieve the main goal of creating an expenses application.
After declaring the example data, you can visualize it on a simple QTableWidget. To do so, you will add this procedure to the Widget constructor.
Warning
Only for the example purpose a QTableWidget will be used, but for more performance-critical applications the combination of a model and a QTableView is encouraged.
1 def __init__(self):
2 super().__init__()
3 self.items = 0
4
5 # Example data
6 self._data = {"Water": 24.5, "Electricity": 55.1, "Rent": 850.0,
7 "Supermarket": 230.4, "Internet": 29.99, "Bars": 21.85,
8 "Public transportation": 60.0, "Coffee": 22.45, "Restaurants": 120}
9
10 # Left
11 self.table = QTableWidget()
12 self.table.setColumnCount(2)
13 self.table.setHorizontalHeaderLabels(["Description", "Price"])
14 self.table.horizontalHeader().setSectionResizeMode(QHeaderView.Stretch)
15
16 # QWidget Layout
17 self.layout = QHBoxLayout(self)
18 self.layout.addWidget(self.table)
19
20 # Fill example data
21 self.fill_table()
As you can see, the code also includes a QHBoxLayout that provides the container to place widgets horizontally.
Additionally, the QTableWidget allows for customizing it, like adding the labels for the two columns that will be used, and to stretch the content to use the whole Widget space.
The last line of code refers to filling the table*, and the code to perform that task is displayed below.
1 def fill_table(self, data=None):
2 data = self._data if not data else data
3 for desc, price in data.items():
4 self.table.insertRow(self.items)
5 self.table.setItem(self.items, 0, QTableWidgetItem(desc))
6 self.table.setItem(self.items, 1, QTableWidgetItem(str(price)))
7 self.items += 1
Having this process on a separate method is a good practice to leave the constructor more readable, and to split the main functions of the class in independent processes.
Right side layout#
Because the data that is being used is just an example, you are required to include a mechanism to input items to the table, and extra buttons to clear the table’s content, and also quit the application.
For input lines along with descriptive labels, you will use a QFormLayout. Then, you will nest the form layout into a QVBoxLayout along with the buttons.
1 # Right
2 self.description = QLineEdit()
3 self.description.setClearButtonEnabled(True)
4 self.price = QLineEdit()
5 self.price.setClearButtonEnabled(True)
6
7 self.add = QPushButton("Add")
8 self.clear = QPushButton("Clear")
9
10 form_layout = QFormLayout()
11 form_layout.addRow("Description", self.description)
12 form_layout.addRow("Price", self.price)
13 self.right = QVBoxLayout()
14 self.right.addLayout(form_layout)
15 self.right.addWidget(self.add)
16 self.right.addStretch()
17 self.right.addWidget(self.clear)
Leaving the table on the left side and these newly included widgets to the right side will be just a matter to add a layout to our main QHBoxLayout as you saw in the previous example:
1 # QWidget Layout
2 self.layout = QHBoxLayout(self)
3 self.layout.addWidget(self.table)
4 self.layout.addLayout(self.right)
The next step will be connecting those new buttons to slots.
Adding elements#
Each QPushButton have a signal called clicked, that is emitted when you click on the button. This will be more than enough for this example, but you can see other signals in the official documentation.
1 # Signals and Slots
2 self.add.clicked.connect(self.add_element)
3 self.clear.clicked.connect(self.clear_table)
As you can see on the previous lines, we are connecting each clicked signal to different slots. In this example slots are normal class methods in charge of perform a determined task associated with our buttons. It is really important to decorate each method declaration with a @Slot(), that way, PySide6 knows internally how to register them into Qt and they will be invokable from Signals of QObjects when connected.
1 @Slot()
2 def add_element(self):
3 des = self.description.text()
4 price = self.price.text()
5
6 self.table.insertRow(self.items)
7 self.table.setItem(self.items, 0, QTableWidgetItem(des))
8 self.table.setItem(self.items, 1, QTableWidgetItem(price))
9
10 self.description.clear()
11 self.price.clear()
12
13 self.items += 1
14
15 def fill_table(self, data=None):
16 data = self._data if not data else data
17 for desc, price in data.items():
18 self.table.insertRow(self.items)
19 self.table.setItem(self.items, 0, QTableWidgetItem(desc))
20 self.table.setItem(self.items, 1, QTableWidgetItem(str(price)))
21 self.items += 1
22
23 @Slot()
24 def clear_table(self):
25 self.table.setRowCount(0)
26 self.items = 0
Since these slots are methods, we can access the class variables, like our QTableWidget to interact with it.
The mechanism to add elements into the table is described as the following:
get the description and price from the fields,
insert a new empty row to the table,
set the values for the empty row in each column,
clear the input text fields,
include the global count of table rows.
To exit the application you can use the quit() method of the unique QApplication instance, and to clear the content of the table you can just set the table row count, and the internal count to zero.
Verification step#
Adding information to the table needs to be a critical action that require a verification step to avoid adding invalid information, for example, empty information.
You can use a signal from QLineEdit called textChanged which will be emitted every time something inside changes, i.e.: each key stroke.
You can connect two different object’s signal to the same slot, and this will be the case for your current application:
1 self.description.textChanged.connect(self.check_disable)
2 self.price.textChanged.connect(self.check_disable)
The content of the check_disable slot will be really simple:
1 @Slot()
2 def check_disable(self, s):
3 enabled = bool(self.description.text() and self.price.text())
4 self.add.setEnabled(enabled)
You have two options, write a verification based on the current value of the string you retrieve, or manually get the whole content of both QLineEdit. The second is preferred in this case, so you can verify if the two inputs are not empty to enable the button Add.
Note
Qt also provides a special class called QValidator that you can use to validate any input.
Empty chart view#
New items can be added to the table, and the visualization is so far OK, but you can accomplish more by representing the data graphically.
First you will include an empty QChartView placeholder into the right side of your application.
1 # Chart
2 self.chart_view = QChartView()
3 self.chart_view.setRenderHint(QPainter.Antialiasing)
Additionally the order of how you include widgets to the right QVBoxLayout will also change.
1 form_layout = QFormLayout()
2 form_layout.addRow("Description", self.description)
3 form_layout.addRow("Price", self.price)
4 self.right = QVBoxLayout()
5 self.right.addLayout(form_layout)
6 self.right.addWidget(self.add)
7 self.right.addWidget(self.plot)
8 self.right.addWidget(self.chart_view)
9 self.right.addWidget(self.clear)
Notice that before we had a line with self.right.addStretch() to fill up the vertical space between the Add and the Clear buttons, but now, with the QChartView it will not be necessary.
Also, you need include a Plot button if you want to do it on-demand.
Full application#
For the final step, you will need to connect the Plot button to a slot that creates a chart and includes it into your QChartView.
1 # Signals and Slots
2 self.add.clicked.connect(self.add_element)
3 self.plot.clicked.connect(self.plot_data)
4 self.clear.clicked.connect(self.clear_table)
5 self.description.textChanged.connect(self.check_disable)
6 self.price.textChanged.connect(self.check_disable)
That is nothing new, since you already did it for the other buttons, but now take a look at how to create a chart and include it into your QChartView.
1 @Slot()
2 def plot_data(self):
3 # Get table information
4 series = QPieSeries()
5 for i in range(self.table.rowCount()):
6 text = self.table.item(i, 0).text()
7 number = float(self.table.item(i, 1).text())
8 series.append(text, number)
9
10 chart = QChart()
11 chart.addSeries(series)
12 chart.legend().setAlignment(Qt.AlignLeft)
13 self.chart_view.setChart(chart)
The following steps show how to fill a QPieSeries:
create a QPieSeries,
iterate over the table row IDs,
get the items at the i position,
add those values to the series.
Once the series has been populated with our data, you create a new QChart, add the series on it, and optionally set an alignment for the legend.
The final line self.chart_view.setChart(chart) is in charge of bringing your newly created chart to the QChartView.
The application will look like this:
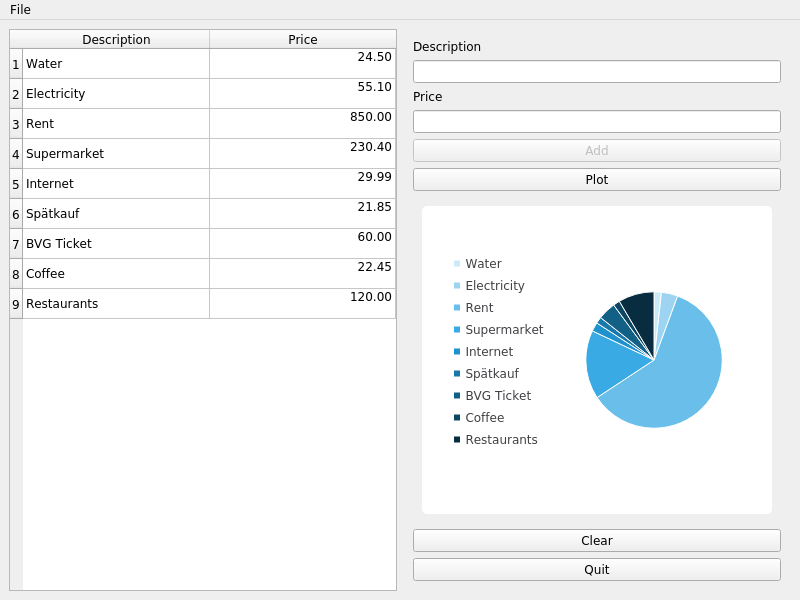
And now you can see the whole code:
1# Copyright (C) 2022 The Qt Company Ltd.
2# SPDX-License-Identifier: LicenseRef-Qt-Commercial
3
4import sys
5from PySide6.QtCore import Qt, Slot
6from PySide6.QtGui import QPainter
7from PySide6.QtWidgets import (QApplication, QFormLayout, QHeaderView,
8 QHBoxLayout, QLineEdit, QMainWindow,
9 QPushButton, QTableWidget, QTableWidgetItem,
10 QVBoxLayout, QWidget)
11from PySide6.QtCharts import QChartView, QPieSeries, QChart
12
13
14class Widget(QWidget):
15 def __init__(self):
16 super().__init__()
17 self.items = 0
18
19 # Example data
20 self._data = {"Water": 24.5, "Electricity": 55.1, "Rent": 850.0,
21 "Supermarket": 230.4, "Internet": 29.99, "Bars": 21.85,
22 "Public transportation": 60.0, "Coffee": 22.45, "Restaurants": 120}
23
24 # Left
25 self.table = QTableWidget()
26 self.table.setColumnCount(2)
27 self.table.setHorizontalHeaderLabels(["Description", "Price"])
28 self.table.horizontalHeader().setSectionResizeMode(QHeaderView.Stretch)
29
30 # Chart
31 self.chart_view = QChartView()
32 self.chart_view.setRenderHint(QPainter.Antialiasing)
33
34 # Right
35 self.description = QLineEdit()
36 self.description.setClearButtonEnabled(True)
37 self.price = QLineEdit()
38 self.price.setClearButtonEnabled(True)
39
40 self.add = QPushButton("Add")
41 self.clear = QPushButton("Clear")
42 self.plot = QPushButton("Plot")
43
44 # Disabling 'Add' button
45 self.add.setEnabled(False)
46
47 form_layout = QFormLayout()
48 form_layout.addRow("Description", self.description)
49 form_layout.addRow("Price", self.price)
50 self.right = QVBoxLayout()
51 self.right.addLayout(form_layout)
52 self.right.addWidget(self.add)
53 self.right.addWidget(self.plot)
54 self.right.addWidget(self.chart_view)
55 self.right.addWidget(self.clear)
56
57 # QWidget Layout
58 self.layout = QHBoxLayout(self)
59 self.layout.addWidget(self.table)
60 self.layout.addLayout(self.right)
61
62 # Signals and Slots
63 self.add.clicked.connect(self.add_element)
64 self.plot.clicked.connect(self.plot_data)
65 self.clear.clicked.connect(self.clear_table)
66 self.description.textChanged.connect(self.check_disable)
67 self.price.textChanged.connect(self.check_disable)
68
69 # Fill example data
70 self.fill_table()
71
72 @Slot()
73 def add_element(self):
74 des = self.description.text()
75 price = float(self.price.text())
76
77 self.table.insertRow(self.items)
78 description_item = QTableWidgetItem(des)
79 price_item = QTableWidgetItem(f"{price:.2f}")
80 price_item.setTextAlignment(Qt.AlignRight)
81
82 self.table.setItem(self.items, 0, description_item)
83 self.table.setItem(self.items, 1, price_item)
84
85 self.description.clear()
86 self.price.clear()
87
88 self.items += 1
89
90 @Slot()
91 def check_disable(self, s):
92 enabled = bool(self.description.text() and self.price.text())
93 self.add.setEnabled(enabled)
94
95 @Slot()
96 def plot_data(self):
97 # Get table information
98 series = QPieSeries()
99 for i in range(self.table.rowCount()):
100 text = self.table.item(i, 0).text()
101 number = float(self.table.item(i, 1).text())
102 series.append(text, number)
103
104 chart = QChart()
105 chart.addSeries(series)
106 chart.legend().setAlignment(Qt.AlignLeft)
107 self.chart_view.setChart(chart)
108
109 def fill_table(self, data=None):
110 data = self._data if not data else data
111 for desc, price in data.items():
112 description_item = QTableWidgetItem(desc)
113 price_item = QTableWidgetItem(f"{price:.2f}")
114 price_item.setTextAlignment(Qt.AlignRight)
115 self.table.insertRow(self.items)
116 self.table.setItem(self.items, 0, description_item)
117 self.table.setItem(self.items, 1, price_item)
118 self.items += 1
119
120 @Slot()
121 def clear_table(self):
122 self.table.setRowCount(0)
123 self.items = 0
124
125
126class MainWindow(QMainWindow):
127 def __init__(self, widget):
128 super().__init__()
129 self.setWindowTitle("Tutorial")
130
131 # Menu
132 self.menu = self.menuBar()
133 self.file_menu = self.menu.addMenu("File")
134
135 # Exit QAction
136 exit_action = self.file_menu.addAction("Exit", self.close)
137 exit_action.setShortcut("Ctrl+Q")
138
139 self.setCentralWidget(widget)
140
141
142if __name__ == "__main__":
143 # Qt Application
144 app = QApplication(sys.argv)
145 # QWidget
146 widget = Widget()
147 # QMainWindow using QWidget as central widget
148 window = MainWindow(widget)
149 window.resize(800, 600)
150 window.show()
151
152 # Execute application
153 sys.exit(app.exec())