QML Application Tutorial#
This tutorial provides a quick walk-through of a python application that loads a QML file. QML is a declarative language that lets you design UIs faster than a traditional language, such as C++. The QtQml and QtQuick modules provides the necessary infrastructure for QML-based UIs.
In this tutorial, you’ll also learn how to provide data from Python as a QML initial property, which is then consumed by the ListView defined in the QML file.
Before you begin, install the following prerequisites:
The PySide6 Python packages.
Qt Creator from https://download.qt.io.
The following step-by-step instructions guide you through application development process using Qt Creator:
Open Qt Creator and select File > New File or Project.. menu item to open following dialog:
Select Qt for Python - Empty from the list of application templates and select Choose.
Give a Name to your project, choose its location in the filesystem, and select Finish to create an empty
main.py
andmain.pyproject
.This should create a
main.py
and`main.pyproject
files for the project.Download
view.qml
andlogo.png
and move them to your project folder.Double-click on
main.pyproject
to open it in edit mode, and appendview.qml
andlogo.png
to the files list. This is how your project file should look after this change:{ "files": ["main.py", "view.qml", "logo.png"] }
Now that you have the necessary bits for the application, import the Python modules in your
main.py
, and download country data and format it:1 2import sys 3import urllib.request 4import json 5from pathlib import Path 6 7from PySide6.QtQuick import QQuickView 8from PySide6.QtCore import QStringListModel, QUrl 9from PySide6.QtGui import QGuiApplication 10 11 12if __name__ == '__main__': 13 14 #get our data 15 url = "http://country.io/names.json" 16 response = urllib.request.urlopen(url) 17 data = json.loads(response.read().decode('utf-8')) 18 19 #Format and sort the data 20 data_list = list(data.values()) 21 data_list.sort()
Now, set up the application window using PySide6.QtGui.QGuiApplication, which manages the application-wide settings.
1 2import sys 3import urllib.request 4import json 5from pathlib import Path 6 7from PySide6.QtQuick import QQuickView 8from PySide6.QtCore import QStringListModel, QUrl 9from PySide6.QtGui import QGuiApplication 10 11 12if __name__ == '__main__': 13 14 #get our data 15 url = "http://country.io/names.json" 16 response = urllib.request.urlopen(url) 17 data = json.loads(response.read().decode('utf-8')) 18 19 #Format and sort the data 20 data_list = list(data.values()) 21 data_list.sort() 22 23 #Set up the application window 24 app = QGuiApplication(sys.argv) 25 view = QQuickView() 26 view.setResizeMode(QQuickView.SizeRootObjectToView)
Note
Setting the resize policy is important if you want the root item to resize itself to fit the window or vice-a-versa. Otherwise, the root item will retain its original size on resizing the window.
You can now expose the
data_list
variable as a QML initial property, which will be consumed by the QML ListView item inview.qml
.1 2import sys 3import urllib.request 4import json 5from pathlib import Path 6 7from PySide6.QtQuick import QQuickView 8from PySide6.QtCore import QStringListModel, QUrl 9from PySide6.QtGui import QGuiApplication 10 11 12if __name__ == '__main__': 13 14 #get our data 15 url = "http://country.io/names.json" 16 response = urllib.request.urlopen(url) 17 data = json.loads(response.read().decode('utf-8')) 18 19 #Format and sort the data 20 data_list = list(data.values()) 21 data_list.sort() 22 23 #Set up the application window 24 app = QGuiApplication(sys.argv) 25 view = QQuickView() 26 view.setResizeMode(QQuickView.SizeRootObjectToView) 27 28 #Expose the list to the Qml code 29 my_model = QStringListModel() 30 my_model.setStringList(data_list) 31 view.setInitialProperties({"myModel": my_model})
Load the
view.qml
to theQQuickView
and callshow()
to display the application window.1 2import sys 3import urllib.request 4import json 5from pathlib import Path 6 7from PySide6.QtQuick import QQuickView 8from PySide6.QtCore import QStringListModel, QUrl 9from PySide6.QtGui import QGuiApplication 10 11 12if __name__ == '__main__': 13 14 #get our data 15 url = "http://country.io/names.json" 16 response = urllib.request.urlopen(url) 17 data = json.loads(response.read().decode('utf-8')) 18 19 #Format and sort the data 20 data_list = list(data.values()) 21 data_list.sort() 22 23 #Set up the application window 24 app = QGuiApplication(sys.argv) 25 view = QQuickView() 26 view.setResizeMode(QQuickView.SizeRootObjectToView) 27 28 #Expose the list to the Qml code 29 my_model = QStringListModel() 30 my_model.setStringList(data_list) 31 view.setInitialProperties({"myModel": my_model}) 32 33 #Load the QML file 34 qml_file = Path(__file__).parent / "view.qml" 35 view.setSource(QUrl.fromLocalFile(qml_file.resolve())) 36 37 #Show the window 38 if view.status() == QQuickView.Error: 39 sys.exit(-1) 40 view.show()
Finally, execute the application to start the event loop and clean up.
1 2import sys 3import urllib.request 4import json 5from pathlib import Path 6 7from PySide6.QtQuick import QQuickView 8from PySide6.QtCore import QStringListModel, QUrl 9from PySide6.QtGui import QGuiApplication 10 11 12if __name__ == '__main__': 13 14 #get our data 15 url = "http://country.io/names.json" 16 response = urllib.request.urlopen(url) 17 data = json.loads(response.read().decode('utf-8')) 18 19 #Format and sort the data 20 data_list = list(data.values()) 21 data_list.sort() 22 23 #Set up the application window 24 app = QGuiApplication(sys.argv) 25 view = QQuickView() 26 view.setResizeMode(QQuickView.SizeRootObjectToView) 27 28 #Expose the list to the Qml code 29 my_model = QStringListModel() 30 my_model.setStringList(data_list) 31 view.setInitialProperties({"myModel": my_model}) 32 33 #Load the QML file 34 qml_file = Path(__file__).parent / "view.qml" 35 view.setSource(QUrl.fromLocalFile(qml_file.resolve())) 36 37 #Show the window 38 if view.status() == QQuickView.Error: 39 sys.exit(-1) 40 view.show() 41 42 #execute and cleanup 43 app.exec() 44 del view
Your application is ready to be run now. Select Projects mode to choose the Python version to run it.
Run the application by using the CTRL+R
keyboard shortcut to see if it
looks like this:
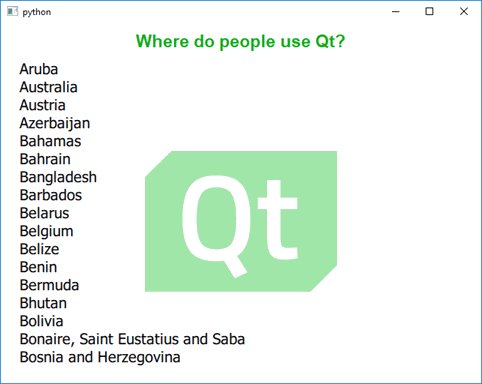
You could also watch the following video tutorial for guidance to develop this application: